SDK Configuration
SDK Configuration
Helpshift provides several config options which can be used to customize behaviour of the SDK. These options are boolean flags which can be passed with the Helpshift APIs such as showConversation, showFAQs etc.
Install Options
enableInAppNotification
Flag | enableInAppNotification |
Values | "yes" / "no" |
Default | "yes" |
If you want to disable the in-app notifications, please set this
variable to "no"
in the config dictionary passed to the install
.
For Example:
HashMap config = new HashMap();
config.put("enableInAppNotification", "yes");
HelpshiftBridge.install(this, // "this" should be the application object
"YOUR_API_KEY",
"<YOUR_HELPSHIFT_DOMAIN>.helpshift.com",
"YOUR_APP_ID",
config);
Customizing notification icons
By default the application icon is used as the notification icon. You can customize the notification icons via the config dictionary passed to the install
call.
For Example:
HashMap config = new HashMap();
config.put("notificationIcon", R.drawable.notification_icon_small);
config.put("largeNotificationIcon", R.drawable.notification_icon_large);
HelpshiftBridge.install(this, // "this" should be the application object
"YOUR_API_KEY",
"<YOUR_HELPSHIFT_DOMAIN>.helpshift.com",
"YOUR_APP_ID",
config);
Customizing notification sound
By default the default device notification sound is used for helpshift notifications. You can customize the notification sound via the config dictionary passed to the install
call.
For Example:
HashMap config = new HashMap();
config.put("notificationSound", R.raw.notification_sound);
HelpshiftBridge.install(this, // "this" should be the application object
"YOUR_API_KEY",
"<YOUR_HELPSHIFT_DOMAIN>.helpshift.com",
"YOUR_APP_ID",
config);
Notification channels
Applicable to SDK v1.8.0 and above.
Starting from Android Oreo, Helpshift notifications will have a default channel set as In-app Support
. The name and description for this default channel can be changed by setting the following settings. If you want to customize the name and description for the default channel, you can do so by using the following resources in your strings.xml file:
<string name="hs__default_notification_channel_name">Example Support Name</string>
<string name="hs__default_notification_channel_desc">Example Support Description</string>
If you want to customize the notification channels, you can create your own custom channels and set their channel IDs in the in the config dictionary passed to the install
corresponsing to following key: supportNotificationChannelId
.
For Example:
HashMap config = new HashMap();
config.put("supportNotificationChannelId", "SUPPORT_CHANNEL_ID");
HelpshiftBridge.install(this, // "this" should be the application object
"YOUR_API_KEY",
"<YOUR_HELPSHIFT_DOMAIN>.helpshift.com",
"YOUR_APP_ID",
config);
enableDefaultFallbackLanguage
Flag | HS_ENABLE_DEFAULT_FALL_BACK_LANGUAGE |
values | "yes" /"no" |
default | "yes" |
You can enable or disable the SDK default fallback language when showing FAQs using this flag. When set to false, the Helpshift SDK will not fallback to the default language that is English, when showing FAQs. This flag is to be passed only in the config for the HelpshiftBridge.install
call.
This configuration does not apply to the QuickSearch Bot suggested FAQs.
For Example:
HashMap config = new HashMap();
config.put("enableDefaultFallbackLanguage", "yes");
HelpshiftBridge.install(this, // "this" should be the application object
"YOUR_API_KEY",
"<YOUR_HELPSHIFT_DOMAIN>.helpshift.com",
"YOUR_APP_ID",
config);
Using Custom Fonts
Applicable to version 1.4.0 and above.
Step 1: Add the font to your Resources/fonts
folder.
Step 2: Pass the font location relative to the Resources
folder as follows during installation. If the font file DancingScript-Regular.ttf
lies in the fonts
folder inside the Resources
folder, then your path is fonts/DancingScript-Regular.ttf
.
For Example:
HashMap config = new HashMap();
config.put("font", "fonts/DancingScript-Regular.ttf");
HelpshiftBridge.install(this, // "this" should be the application object
"YOUR_API_KEY",
"<YOUR_HELPSHIFT_DOMAIN>.helpshift.com",
"YOUR_APP_ID",
config);
Step 3: Test the font in simulator. Errors in font names are ignored by the SDK —
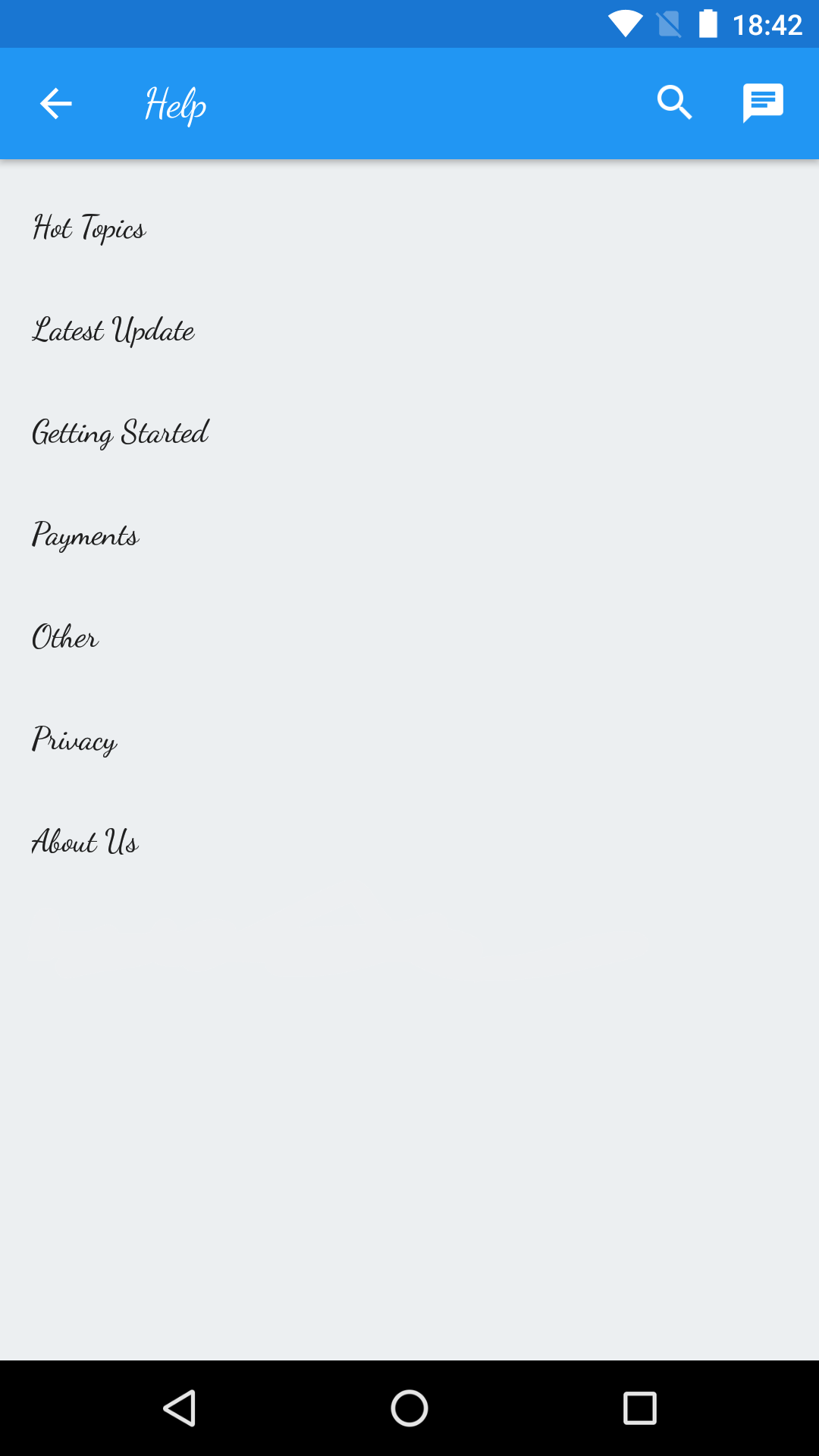
screenOrientation
Applicable to version 1.6.0 and above
The screen orientation of Helpshift SDK screens can be fixed by setting the screenOrientation to constants available in the ActivityInfo class.
For example, you may want to fix the orientation to ActivityInfo.SCREEN_ORIENTATION_PORTRAIT
for mobile users and ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE
for tablet users.
Flag | screenOrientation |
values | integer values for orientation from ActivityInfo class |
default | ActivityInfo.SCREEN_ORIENTATION_UNSPECIFIED |
For Example:
HashMap config = new HashMap();
config.put("screenOrientation", ActivityInfo.SCREEN_ORIENTATION_SENSOR_LANDSCAPE);
HelpshiftBridge.install(this, // "this" should be the application object
"YOUR_API_KEY",
"<YOUR_HELPSHIFT_DOMAIN>.helpshift.com",
"YOUR_APP_ID",
config);
Due to a bug in Android OS 8.0, setting the requested orientation when opening the Helpshift activity causes a crash. This happens when the targetSDKVersion is greater than 26 and the Activity is not full screen. As a workaround, apps need to set Helpshift's ParentActivity to full screen mode, which will prevent the exception.
enableLogging
Upon setting enableLogging to "yes", Helpshift SDK logs will be generated in the Android logcat. These will be useful for debugging the SDK during integration. Turning on this logging could help the developer resolve common integration issues.
Flag | enableLogging |
values | "yes" /"no" |
default | "no" |
For Example:
HashMap config = new HashMap();
config.put("enableLogging", "yes");
HelpshiftBridge.install(this, // "this" should be the application object
"YOUR_API_KEY",
"<YOUR_HELPSHIFT_DOMAIN>.helpshift.com",
"YOUR_APP_ID",
config);
disableErrorReporting
Disables reporting of Helpshift SDK’s internal error logs. The Helpshift SDK will collect internal error logs and report them to our systems to ensure that we become aware of runtime crashes and we have enough information to fix them.
Flag | disableErrorReporting |
values | "yes" /"no" |
default | "no" |
For Example:
HashMap config = new HashMap();
config.put("disableErrorReporting", "yes");
HelpshiftBridge.install(this, // "this" should be the application object
"YOUR_API_KEY",
"<YOUR_HELPSHIFT_DOMAIN>.helpshift.com",
"YOUR_APP_ID",
config);
API Options
enableContactUs
Flag | enableContactUs |
Values | HS_ENABLE_CONTACT_US_ALWAYS /HS_ENABLE_CONTACT_US_NEVER /HS_ENABLE_CONTACT_US_AFTER_VIEWING_FAQS |
Default | HS_ENABLE_CONTACT_US_ALWAYS |
The enableContactUs
flag controls the visibility of Contact Us
button -
HS_ENABLE_CONTACT_US_ALWAYS | show in the navigation bar, search, and after marking an FAQ unhelpful. |
HS_ENABLE_CONTACT_US_AFTER_VIEWING_FAQS | show only while searching, on FAQ screen and after marking an FAQ unhelpful. |
HS_ENABLE_CONTACT_US_NEVER | do not show "Contact Us" button anywhere in the SDK. |
HS_ENABLE_CONTACT_US_AFTER_MARKING_ANSWER_UNHELPFUL | do not show "Contact Us" button until the user specifically marks an FAQ as unhelpful. |
To override the default value you can pass the arguments in the options dictionary.
Example:
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
ValueMap config;
config[HS_ENABLE_CONTACT_US] = HS_ENABLE_CONTACT_US_NEVER;
HelpshiftCocos2dx::showFAQs(config);
cocos2d::CCDictionary *config = new cocos2d::CCDictionary();
CCString *enableContactUs = new CCString(HS_ENABLE_CONTACT_US_NEVER);
config->setObject(enableContactUs, HS_ENABLE_CONTACT_US);
HelpshiftCocos2dx::showFAQs(config);
This flag has no effect for the showConversation
API call, since there is no Contact Us
button in the conversation screen.
By using this option, you encourage users to read and search FAQs first instead of directly filing new tickets. This facilitates ticket avoidance.
gotoConversationAfterContactUs
This API is now deprecated with Cocos2d-x SDK v4.0.0. Read more about the new conversational experience here
Flag | gotoConversationAfterContactUs |
Values | "yes" / "no" |
Default | "no" |
The gotoConversationAfterContactUs
flag will determine whether the
user lands up in the conversation screen after starting a new
conversation via "Contact Us". This only makes sense if the
enableContactUs
flag takes on the default value.
Example:
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
ValueMap config;
config["gotoConversationAfterContactUs"] = Value("yes");
HelpshiftCocos2dx::showFAQs(config);
cocos2d::CCDictionary *config = new cocos2d::CCDictionary();
config->setObject(new CCString("yes"), HS_GOTO_CONVERSATION_AFTER_CONTACT_US);
HelpshiftCocos2dx::showFAQs(config);
For showFAQs
, showFAQSection
and showSingleFAQ
, setting gotoConversationAfterContactUs
makes sense only if enableContactUs
is yes
.
requireEmail
This API is now deprecated with Cocos2d-x SDK v4.0.0. Read more about the new conversational experience here
Flag | requireEmail |
Values | "yes" / "no" |
Default | "no" |
The requireEmail
flag will determine whether email is required or optional for starting a new conversation.
When the flag is set to yes
customer's email will be required. If set to no
email will be optional.
For example:
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
ValueMap config;
config["requireEmail"] = Value("yes");
HelpshiftCocos2dx::showFAQs(config);
cocos2d::CCDictionary *config = new cocos2d::CCDictionary();
CCString *requireEmail = new CCString("yes");
config->setObject(requireEmail, HS_REQUIRE_EMAIL);
HelpshiftCocos2dx::showFAQs(config);
If "New Issue Forwarding" is ON or if "Allow anonymous issues" is ON in the agent dashboard, then this flag will be ignored.
Once requireEmail
flag is set, the SDK will use that value for all new conversations until it is changed again.
hideNameAndEmail
- This API is now deprecated with Cocos2d-x SDK v4.0.0. Read more about the new conversational experience here
Flag | hideNameAndEmail |
Values | "yes" / "no" |
Default | "no" |
The hideNameAndEmail
flag will hide the name and email fields when the user starts a new conversation.
When the flag is set to yes
the name and email fields will be hidden. If set to no
the default behaviour will resume.
If "New Issue Forwarding" is ON then this flag will be ignored when name and email are not available to the SDK. You can use the setNameAndEmail
API, to supply the SDK with name and email in this case.
If "Allow anonymous issues" is ON, under app settings in the agent dashboard, then this flag will be ignored.
Also, if the requireEmail
flag is set to no
and email is not available to the SDK, then hideNameAndEmail
flag will be ignored.
For example:
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
ValueMap config;
config["hideNameAndEmail"] = Value("yes");
HelpshiftCocos2dx::showFAQs(config);
cocos2d::CCDictionary *config = new cocos2d::CCDictionary();
CCString *hideNameEmail = new CCString("yes");
config->setObject(hideNameEmail, HS_HIDE_NAME_AND_EMAIL);
HelpshiftCocos2dx::showFAQs(config);
Once hideNameAndEmail
flag is set, the SDK will use that value for all new conversations until it is changed again.
conversationPrefillText
Flag | conversationPrefillText |
Values | Non-empty string |
The conversationPrefillText
API option will prefill a new conversation description, with the supplied string.
This is useful where you might want your users to send you diagnostic information in the conversation description, for example if the app hits an exception, etc.
Example:
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
ValueMap config;
config[HS_CONVERSATION_PREFILL_TEXT] = Value("This is prefilled text");
HelpshiftCocos2dx::showConversation(config);
cocos2d::CCDictionary *config = new cocos2d::CCDictionary();
CCString *prefill = new CCString("This is prefilled");
config->setObject(prefill, HS_CONVERSATION_PREFILL_TEXT);
HelpshiftCocos2dx::showConversation(config);
The conversationPrefillText
option takes effect only for the showConversation
API.
enableFullPrivacy
Flag | enableFullPrivacy |
Values | "yes" / "no" |
Default | "no" |
In scenarios where the user attaches objectionable content in the screenshots, it becomes a huge COPPA
concern. The enableFullPrivacy option will help solve this problem.
If enableFullPrivacy option is set to "yes"
, HS SDK does the following:
- Disables user-initiated screenshots - players cannot send images without being requested by an Agent
- Does not collect any of the following personal information:
Map with "private-data" key inside custom meta-data.
Country code.
Carrier name.
To send personally identifiable information through custom meta-data, the information must be added inside a dictionary with a "private-data" key. If this option is set to "yes"
, this data will be removed when the user starts a new conversation.
Example:
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
ValueMap config;
config["enableFullPrivacy"] = Value("yes");
HelpshiftCocos2dx::showConversation(config);
cocos2d::CCDictionary *config = new cocos2d::CCDictionary();
CCString *enableFullPrivacy = new CCString("yes");
config->setObject(enableFullPrivacy, HS_ENABLE_FULL_PRIVACY);
HelpshiftCocos2dx::showConversation(config);
Best Practices
In your registration process, ask your user for his/her age. If the user's age is 13 or younger, set enableFullPrivacy to "yes"
. This way, you comply with COPPA for your children users, but collect valuable user and device data for your other users.
- Once enableFullPrivacy flag is set, the SDK will use that value for all further sessions until it is changed again.
- For SDK versions 1.6.0 and below, if the enableFullPrivacy flag is set, the email collection requirement is always set to optional. If requiredEmail is true, enableFullPrivacy will override this configuration and set the email requirement as optional. For hiding name and email for all users, developers enable a Dashboard configuration named "Allow anonymous issues".
- For SDK versions 1.7.0 and above, if the enableFullPrivacy flag is set, email and name will not be collected and the fields will be hidden. enableFullPrivacy will override all other flags pertaining to name and email fields. This will allow developers to hide name and email for a specific set of users exposed to the enableFullPrivacy flag.
- For Cocos2d-x SDK versions 4.0.0 and above, this flag is ignored in the flows created to show Dynamic forms. Following flows do not honor this flag
ConversationFlow
FAQsFlow
SingleFAQFlow
FAQSectionFlow
CustomFlow
showSearchOnNewConversation
- This API is now deprecated with Cocos2d-x SDK v4.0.0. Read more about the new conversational experience here
Flag | showSearchOnNewConversation |
Values | "yes" / "no" |
Default | "no" |
If showSearchOnNewConversation flag is set to yes
, the user will be taken to a view which shows the search results relevant to the conversation text that he has entered upon clicking the 'Send' button. This is to avoid tickets which are already answered in the FAQs. The user will still be able to start a new conversation with the same text. He can also go through one of the FAQs and find a solution to his query, and exit the session without submitting a ticket.
Default value is no
, ie., this feature will not be enabled unless you explicitly pass yes
for this flag.
Example:
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
ValueMap config;
config[HS_SHOW_SEARCH_ON_NEW_CONVERSATION] = Value("yes");
HelpshiftCocos2dx::showConversation(config);
cocos2d::CCDictionary *config = new cocos2d::CCDictionary();
CCString *showSearchOnNewConversation = new CCString("yes");
config->setObject(showSearchOnNewConversation, HS_SHOW_SEARCH_ON_NEW_CONVERSATION);
HelpshiftCocos2dx::showConversation(config);
The conversationPrefillText
option takes effect only for the showConversation
API.
showConversationResolutionQuestion
For v4.0+
This SDK configuration is deprecated with v4.0+ and default value will be treated as False.
If you want to use this feature, you can turn the feature on and off ("Resolution Question") from the In-App Configuration page.(Settings > App settings)
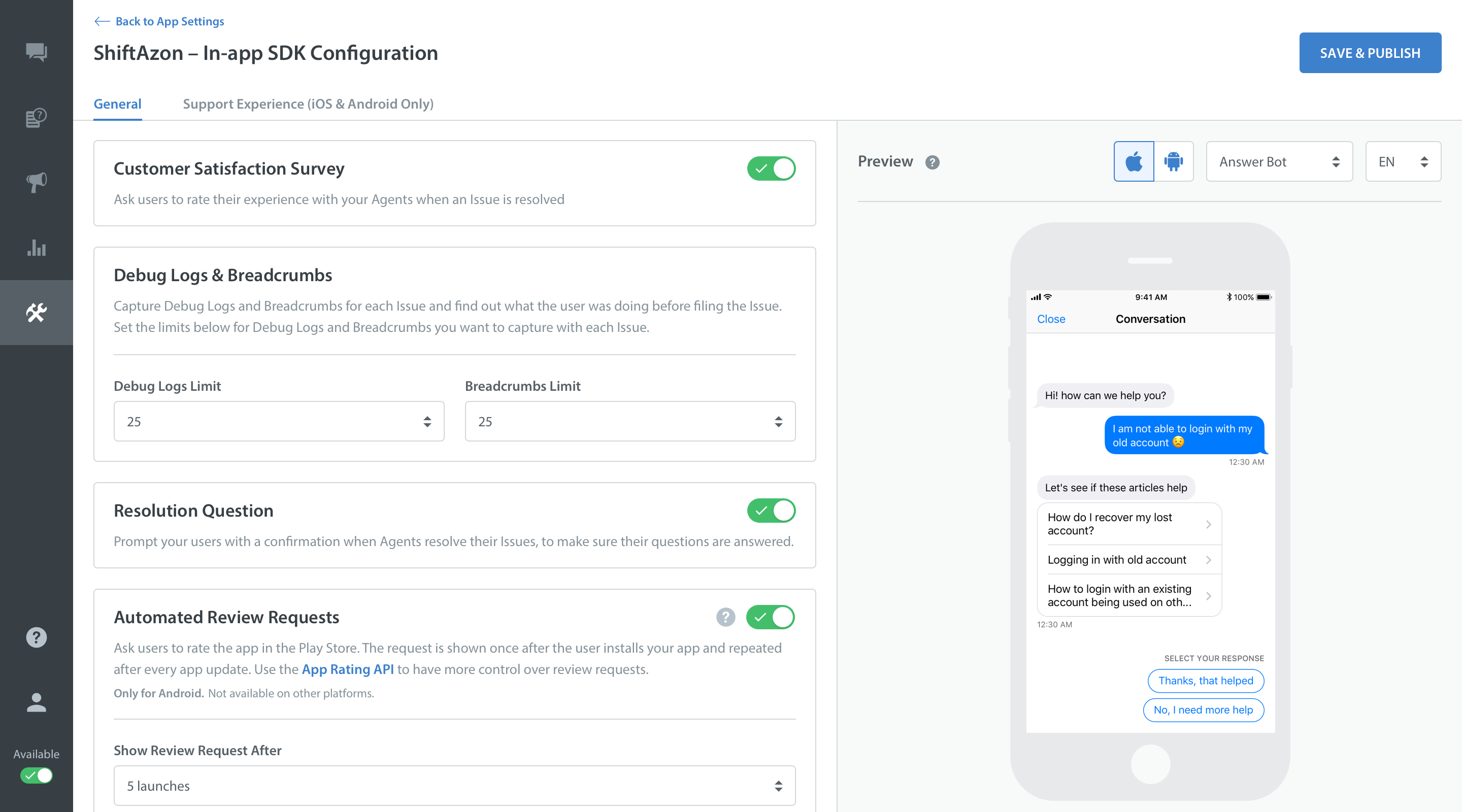
For Older Cocos2d-x SDK versions 1.9.0 and below, please read the following:
Flag | HS_SHOW_CONVERSATION_RESOLUTION_QUESTION |
Values | "yes" /"no" |
Default | "yes" |
By default the Helpshift SDK will show the conversation resolution question to the user, to confirm if the conversation was resolved. On resolving the conversation from the admin dashboard will now take the user directly to the "Start a new conversation" state. If you want to disable the conversation resolution question, set showConversationResolutionQuestion
to no
.
Default value is yes
, ie., this feature will not be disabled unless you explicitly pass false
for this flag.
Example :
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
ValueMap config;
config[HS_SHOW_CONVERSATION_RESOLUTION_QUESTION] = Value("yes")
HelpshiftCocos2dx::showConversation(config);
cocos2d::CCDictionary *config = new cocos2d::CCDictionary();
CCString *showConversationResolutionQuestion = new CCString("yes");
config->setObject(showConversationResolutionQuestion, HS_SHOW_CONVERSATION_RESOLUTION_QUESTION);
HelpshiftCocos2dx::showConversation(config);
customContactUsFlows
Applicable to Cocos2d-x plugin version 1.2.0 and above.
Option | customContactUsFlows |
Value | List of Flows. Flows can be created by using the Guided Issue Filing APIs |
Default | There is no default value for this configuration. |
This configuration allows you to override the Contact Us buttons inside the Helpshift SDK and show Guided Issue Filing when a user taps on the Contact Us buttons.
Example :
Let's say you want the users to see a particular FAQ section, a single FAQ and 2 different Contact Us flows with different prefill texts when they tap the Contact Us buttons within the Helpshift SDK. In this case, you would configure the menuHelpCallback
(a button which triggers Helpshift SDK) in the following way :
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
void AppDelegate::menuHelpCallback(Ref* pSender) {
ValueVector forms;
ValueMap faqSectionFlow;
faqSectionFlow[HS_FLOW_TYPE] = HS_FAQ_SECTION_FLOW;
faqSectionFlow[HS_FLOW_TITLE] = Value("FAQ Section");
faqSectionFlow[HS_FLOW_DATA] = "1";
forms.push_back(Value(faqSectionFlow));
ValueMap singleFAQFlow;
singleFAQFlow[HS_FLOW_TYPE] = HS_SINGLE_FAQ_FLOW;
singleFAQFlow[HS_FLOW_TITLE] = Value("Single FAQ");
singleFAQFlow[HS_FLOW_DATA] = "2";
forms.push_back(Value(singleFAQFlow));
ValueMap conversationFlow1;
conversationFlow1[HS_FLOW_TYPE] = HS_CONVERSATION_FLOW;
conversationFlow1[HS_FLOW_TITLE] = Value("Contact us about our app");
forms.push_back(Value(conversationFlow1));
ValueMap conversationFlow2;
conversationFlow2[HS_FLOW_TYPE] = HS_CONVERSATION_FLOW;
conversationFlow2[HS_FLOW_TITLE] = Value("Contact us about our in-app purchase");
forms.push_back(Value(conversationFlow2));
ValueMap showFAQsConfig;
showFAQsConfig[HS_CUSTOM_CONTACT_US_FLOWS] = Value(forms);
showFAQsConfig[HS_ENABLE_CONTACT_US] = Value("yes");
HelpshiftCocos2dx::showFAQs(showFAQsConfig);
}
void AppDelegate::menuHelpCallback(Ref* pSender) {
cocos2d::CCArray *forms = CCArray::create();
cocos2d::CCDictionary *singleSectionForm = CCDictionary::create();
singleSectionForm->setObject(new CCString(HS_SINGLE_FAQ_FLOW), HS_FLOW_TYPE);
singleSectionForm->setObject(new CCString("Single Section"), HS_FLOW_TITLE);
singleSectionForm->setObject(new CCString("95"), HS_FLOW_DATA);
forms->addObject(singleSectionForm);
cocos2d::CCDictionary *singleFAQForm = CCDictionary::create();
singleFAQForm->setObject(new CCString(HS_FAQ_SECTION_FLOW), HS_FLOW_TYPE);
singleFAQForm->setObject(new CCString("Single FAQ"), HS_FLOW_TITLE);
singleFAQForm->setObject(new CCString("9"), HS_FLOW_DATA);
forms->addObject(singleFAQForm);
cocos2d::CCDictionary *conversationFlow1 = CCDictionary::create();
conversationFlow1->setObject(new CCString(HS_CONVERSATION_FLOW), HS_FLOW_TYPE);
conversationFlow1->setObject(new CCString("Contact us about our app"), HS_FLOW_TITLE);
forms->addObject(conversationFlow1);
cocos2d::CCDictionary *conversationFlow2 = CCDictionary::create();
conversationFlow2->setObject(new CCString(HS_CONVERSATION_FLOW), HS_FLOW_TYPE);
conversationFlow2->setObject(new CCString("Contact us about our
in-app purchase"), HS_FLOW_TITLE);
forms->addObject(conversationFlow2);
cocos2d::CCDictionary *showFAQsConfig = CCDictionary::create();
showFAQsConfig->setObect(forms, HS_CUSTOM_CONTACT_US_FLOWS);
HelpshiftCocos2dx::showFAQs(showFAQsConfig);
}
Anytime the Helpshift SDK is presented via menuHelpCallback
with this configuration, the Contact Us buttons in the SDK will redirect to Guided Issue Filing with the flows provided in the HS_CUSTOM_CONTACT_US_FLOWS
list.
Once a particular flow is selected, the subsequent Contact Us buttons will not redirect to Guided Issue Filing again. They will function as normal Contact Us buttons. This is to avoid an infinite loop of Guided Issue Filing screens.
Custom flows can be nested by passing another custom flows configuration while creating a flow.
Example :
Let's say you want to configure singleFAQFlow
in the above example to show showConversationFlow1
and showConversationFlow2
through the Contact Us buttons inside it. In this case, you would configure the menuHelpCallback
button (a button which triggers Helpshift SDK) in the following way :
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
void AppDelegate::menuHelpCallback(Ref* pSender) {
ValueVector forms;
ValueMap faqSectionFlow;
faqSectionFlow[HS_FLOW_TYPE] = HS_FAQ_SECTION_FLOW;
faqSectionFlow[HS_FLOW_TITLE] = Value("FAQ Section");
faqSectionFlow[HS_FLOW_DATA] = "1";
forms.push_back(Value(faqSectionFlow));
ValueVector nestedCustomContactUsFlows;
ValueMap conversationFlow1;
conversationFlow1[HS_FLOW_TYPE] = HS_CONVERSATION_FLOW;
conversationFlow1[HS_FLOW_TITLE] = Value("Contact us about our app");
nestedCustomContactUsFlows.push_back(Value(conversationFlow1));
ValueMap conversationFlow2;
conversationFlow2[HS_FLOW_TYPE] = HS_CONVERSATION_FLOW;
conversationFlow2[HS_FLOW_TITLE] = Value("Contact us about our in-app purchase");
nestedCustomContactUsFlows.push_back(Value(conversationFlow2));
ValueMap singleFAQFlowConfig;
singleFAQFlowConfig[HS_CUSTOM_CONTACT_US_FLOWS] = Value(nestedCustomContactUsFlows);
ValueMap singleFAQFlow;
singleFAQFlow[HS_FLOW_TYPE] = HS_SINGLE_FAQ_FLOW;
singleFAQFlow[HS_FLOW_TITLE] = Value("Single FAQ");
singleFAQFlow[HS_FLOW_DATA] = "2";
singleFAQFlow[HS_FLOW_CONFIG] = Value(singleFAQFlowConfig);
forms.push_back(Value(singleFAQFlow));
ValueMap showFAQsConfig;
showFAQsConfig[HS_CUSTOM_CONTACT_US_FLOWS] = Value(forms);
HelpshiftCocos2dx::showFAQs(showFAQsConfig);
}
void AppDelegate::menuHelpCallback(Ref* pSender) {
cocos2d::CCArray *forms = CCArray::create();
cocos2d::CCDictionary *faqSectionFlow = CCDictionary::create();
faqSectionFlow->setObject(new CCString(HS_FAQ_SECTION_FLOW), HS_FLOW_TYPE);
faqSectionFlow->setObject(new CCString("FAQ Section"), HS_FLOW_TITLE);
faqSectionFlow->setObject(new CCString("9"), HS_FLOW_DATA);
forms->addObject(faqSectionFlow);
cocos2d::CCArray *nestedCustomContactUsFlows = CCArray::create();
cocos2d::CCDictionary *conversationFlow1 = CCDictionary::create();
conversationFlow1->setObject(new CCString(HS_CONVERSATION_FLOW), HS_FLOW_TYPE);
conversationFlow1->setObject(new CCString("Contact us about our app"), HS_FLOW_TITLE);
nestedCustomContactUsFlows->addObject(conversationFlow1);
cocos2d::CCDictionary *conversationFlow2 = CCDictionary::create();
conversationFlow2->setObject(new CCString(HS_CONVERSATION_FLOW), HS_FLOW_TYPE);
conversationFlow2->setObject(new CCString("Contact us about our in-app purchase"), HS_FLOW_TITLE);
nestedCustomContactUsFlows->addObject(conversationFlow2);
cocos2d::CCDictionary *singleFAQFlowConfig = CCDictionary::create();
singleFAQFlowConfig->setObject(nestedCustomContactUsFlows, HS_CUSTOM_CONTACT_US_FLOWS);
cocos2d::CCDictionary *singleFAQFlow = CCDictionary::create();
singleFAQFlow->setObject(new CCString(HS_SINGLE_FAQ_FLOW), HS_FLOW_TYPE);
singleFAQFlow->setObject(new CCString("Single FAQ"), HS_FLOW_TITLE);
singleFAQFlow->setObject(new CCString("9"), HS_FLOW_DATA);
singleFAQFlow->setObject(singleFAQFlowConfig, HS_FLOW_CONFIG);
forms->addObject(singleFAQFlow);
cocos2d::CCDictionary *showFAQsConfig = CCDictionary::create();
showFAQsConfig->setObject(forms, HS_CUSTOM_CONTACT_US_FLOWS);
HelpshiftCocos2dx::showFAQs(showFAQsConfig);
}
With this configuration, the Contact Us buttons in singleFAQFlow
View will redirect to Guided Issue Filing with showConversationFlow1
and showConversationFlow2
.
This configuration will work only when there is no open conversation for the user, otherwise every Contact Us button will transition to the chat screen.
showConversationInfoScreen
Applicable to version 1.6.0 and above.
Flag | showConversationInfoScreen |
Values | "yes" /"no" |
Default | "no" |
The showConversationInfoScreen
flag will determine if users can see the Conversation info screen for an ongoing Conversation. If set to "yes"
, the Conversation info icon will be shown in the active Conversation screen. Clicking on it will show the Conversation info screen.
Example :
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
ValueMap config;
config[HS_SHOW_CONVERSATION_INFO_SCREEN] = Value("yes")
HelpshiftCocos2dx::showConversation(config);
cocos2d::CCDictionary *config = new cocos2d::CCDictionary();
CCString *showConversationInfoScreen = new CCString("yes");
config->setObject(showConversationInfoScreen, HS_SHOW_CONVERSATION_INFO_SCREEN);
HelpshiftCocos2dx::showConversation(config);
The info screen would only be visible when an QuickSearch Bot/Identity Bot (if configured) complete their tasks and Automations/Agents can act on the new conversation.
Minimum issue description length
You can set the minimum length requirement of text for user while filing the new issue using the integer resource hs__issue_description_min_chars
. It's default value is set to 1 for all languages and it can be configured per language.
If number of characters are less than the defined minimum length requirement then an error is displayed to the user.
Example :
If you want to have minimum length as 5 characters for Korean language and 10 characters for all other languages then you can achieve this as shown below:
Put the following integer resource in integers.xml
of values-ko
resource directory
<integer name="hs__issue_description_min_chars">5</integer>
and the following integer resource in integers.xml
of values
resource directory
<integer name="hs__issue_description_min_chars">10</integer>
enableTypingIndicator
The typing indicator can only be enabled for Issues filed with SDK v1.7.0 and above.
A graphical typing indicator is shown to the end user if an Agent is currently replying to the same Conversation. The HS_ENABLE_TYPING_INDICATOR
flag will enable/disable the aforementioned indicator.
- This SDK configuration has been deprecated since this configuration has shifted to the In-App SDK configuration page. (Settings>App settings)
- For Cocos2d-x SDK v4.0 and above, Typing Indicator configuration ("Show Agent Typing Indicator") is a part of In-app SDK configurations page.
- If configuration is turned ON from the configurations page OR SDK configuration is used, Typing Indicator will be shown to the end users.
+/ .full-width /
Flag | HS_ENABLE_TYPING_INDICATOR |
Values | "yes"/"no" |
Default | "no" |
For Example:
- For Cocos2d-x 3.x
- For Cocos2d-x 2.x :
ValueMap showConversationConfig;
showConversationConfig[HS_ENABLE_TYPING_INDICATOR] = Value("yes");
HelpshiftCocos2dx::showConversation(showConversationConfig);
cocos2d::CCDictionary *config = CCDictionary::create();
CCString *enableTypingIndicator = CCString::create("yes");
config->setObject(enableTypingIndicator, HS_ENABLE_TYPING_INDICATOR);
HelpshiftCocos2dx::showConversation(config);
Configuration Summary
Option / API | showFAQs | showFAQSection | showSingleFAQ | showConversation |
---|---|---|---|---|
enableContactUs | Supported | Supported | Supported | Not Supported |
enableFullPrivacy | Supported | Supported | Supported | Supported |
showConversationResolutionQuestion | Supported | Supported | Supported | Supported |
customContactUsFlows | Supported | Supported | Supported | Not Supported |
showConversationInfoScreen | Supported | Supported | Supported | Supported |
enableTypingIndicator | Supported | Supported | Supported | Supported |