Getting Started
You're 3 steps away from adding great in-app support to your React Native application.
Guide to integrating the React Native plugin for the Helpshift SDK X. You can also refer helpshift-plugin-sdkx-react-native
package here.
Requirements
- Node v18 or above
- Android Studio
- Xcode 12 or above
- Java Development Kit
- Supported Android OS versions:
- SDK X is functional only above API Level 24 i.e Android OS 7 Nougat and above.
- The minSDKVersion in code is kept as API Level 16 to allow importing the SDK and compiling in apps that allow installation till API Level 16 i.e Android 4.4 Jelly Bean.
- If your app is installed on a device below API level 24 (i.e below Android OS 7 Nougat) then you can choose to have a different medium for providing support. For example, Email, Web etc. We log an UnsupportedOSVersionException if the SDK is initialized below API Level 24.
- Supported iOS versions: iOS 13 and above
- For details regarding Helpshift SDK's support policy, please refer to this article.
With 10.3.1 release, react native version support has been upgraded to v0.70.15 & above.
Installation
Open a Terminal in your project's folder and run:
- npm
- yarn
$ npm i helpshift-plugin-sdkx-react-native
$ yarn add helpshift-plugin-sdkx-react-native
- Additionally, for the iOS platform, there is a requirement to link the native parts of the library
$ npx pod-install
- After that, re-run your react application and start using Helpshift SDKX functions.
Here, You should verify that helpshift-plugin-sdkx-react-native
has been added to package.json
"dependencies": {
.....
"helpshift-plugin-sdkx-react-native": <plugin-sdkx-version>,
.....
},
For iOS: Info.plist changes: Addition of usage description strings
When the “User Attachments” feature is enabled from the In-app SDK Configuration section, the SDK allows the user to upload the attachments. The attachments can be picked up from the Photo Library or Files. The options for these sources look like:
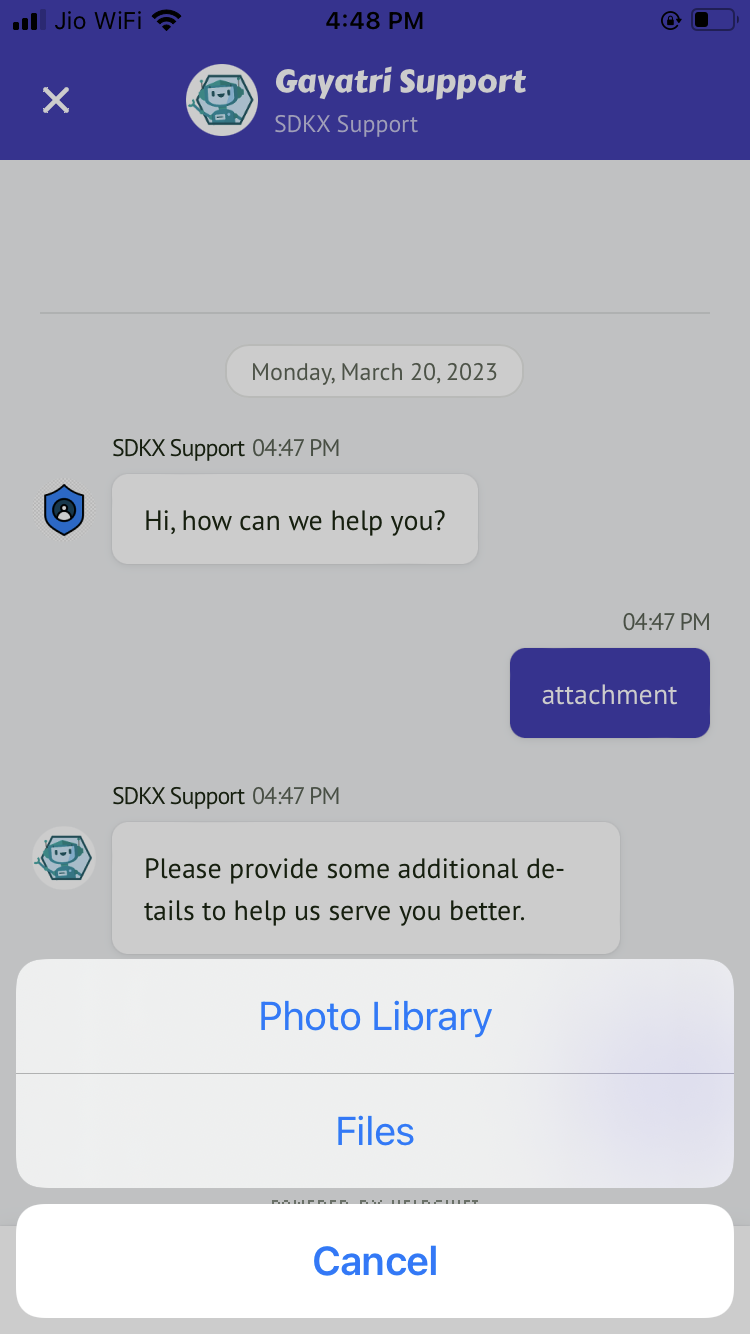
To use the photo library, Apple requires the app to have Usage Description strings in the Info.plist while. Failing to add these strings might result in app rejection. The following strings are needed for the attachment feature:
Key | Suggested string | Notes |
---|---|---|
NSPhotoLibraryUsageDescription | “We need to access photos library to allow users manually pick images meant to be sent as an attachment for help and support reasons.” | This is not needed if your app is iOS 11 or above. Below iOS 11, this key is compulsory else the app may crash when user tries to open the photo library for attaching photos. |
Note that the above strings are just a suggested description. If you need localisations for the same, please Contact Us
Initializing Helpshift in your app
First, create an app on the Helpshift Dashboard
Create an app with Android and iOS as selected
Platform
Helpshift uniquely identifies each registered App with a combination of 2 tokens:
Domain Name | Your Helpshift domain. E.g. happyapps.helpshift.com |
Platform ID | Your App's unique Platform ID (App's App ID on dashboard is your Platform ID) |
You can find these by navigating to Settings
>SDKs (for Developers)
in your agent dashboard.
Select your App and check Android
and iOS
as a platform from the dropdowns and copy
the 2 tokens to be passed when initializing Helpshift.
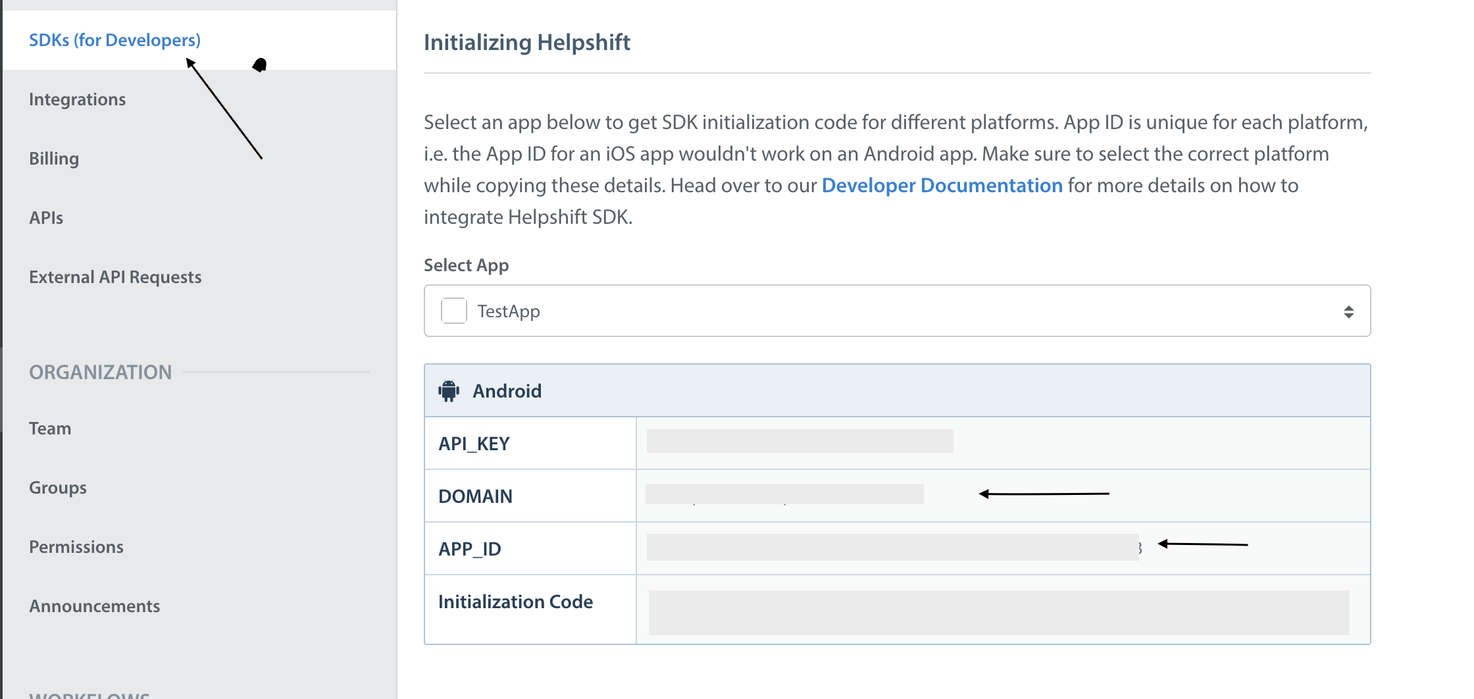
Initialize SDK by calling install
method before any other interaction with the Helpshift SDK in App.tsx
.(It should be used in the useEffect
hook in react native call). For more details click here
Initialize
import {
install
} from 'helpshift-plugin-sdkx-react-native';
let installConfig = {
'enableLogging': true,
'manualLifecycleTracking': false
}
let appId = Platform.select({
android: <Android_Platform_ID>,
ios: <iOS_Platform_ID>,
});
if (appId != null) {
install(appId, <domain>, installConfig);
} else {
//Display your error here.
}
Start using Helpshift
Helpshift is now integrated with your app. You should now use the support APIs for conversation screens inside your app.
Since the Helpshift plugin is meant for mobile devices only, you should put all Helpshift calls inside conditional checks to make sure they are only called when running on a device.