Getting Started iOS
You're 3 steps away from adding great in-app support to your Unity game.
Guide to integrating the Unity plugin for the Helpshift SDK X which you can call from your C# and Javascript game scripts.
Requirements
- Unity 2018.1.0 and above.
- Xcode 12.0 and above.
- iOS 13 and above.
Download HelpshiftX Unity SDK for iOS
HelpshiftX SDK .zip folder includes:
helpshiftX-plugin-unity-v10.3.0.unitypackage | Unity package of Helpshift SDK X |
unity-jar-resolver (v1.2.170.0) | Resolves Android Helpshift package support lib dependencies. |
Add Helpshift to your Unity project
- Unzip the HelpshiftX Unity SDK package.
- HelpshiftX Unity SDK appears as a standard
.unitypackage
which you can import through the Unity package import procedure. - Following are the steps to import the
helpshiftX-plugin-unity-version.unitypackage
into your Unity game:- In the Open Unity project, navigate to Assets drop-down menu and select the Import Package > Custom Package
- From the unzipped SDK, select
helpshiftX-plugin-unity-version.unitypackage
file to import the Helpshift SDK. - Click Import.
Info.plist changes - Addition of usage description strings
When the “User Attachments” feature is enabled from the In-app SDK Configuration section, the SDK allows the user to upload attachments. The attachments are picked either from the Photo Library of File picker.
For photo library, Apple requires the app to have Photo Library Usage Description string in the Info.plist while. Failing to add these strings might result in app rejection. The following string is needed for the attachment feature:
Key | Suggested string | Notes |
---|---|---|
NSPhotoLibraryUsageDescription | “We need to access photos library to allow users manually pick images meant to be sent as an attachment for help and support reasons.” | This is not needed if your app is iOS 14 or above. Below iOS 14, this key is compulsory else the app may crash when user tries to open the photo library for attaching photos. |
The following instructions apply only to versions below SDK X 10.2.0.
Along with NSPhotoLibraryUsageDescription
(as mentioned above), also add NSCameraUsageDescription
and NSMicrophoneUsageDescription
key in your application's Info.plist file.
Key | Suggested string | Notes |
---|---|---|
NSCameraUsageDescription | “We need to access the camera to allow users to click images meant to be sent as an attachment for help and support reasons.” | This key is needed for capturing a photo using camera and attaching it. |
NSMicrophoneUsageDescription | “We need to access the microphone to allow users to record videos using camera meant to be sent as an attachment for help and support reasons.” | This key is needed for capturing a video using camera and attaching it. |
Note that the above strings are just a suggested description. If you need localisations for the same, please Contact Us.
Initializing Helpshift in your app
Helpshift's namespace
To use Helpshift's APIs, please import the Helpshift's namespace like below
using Helpshift;
First, create an app on the Helpshift Dashboard
Create an app with iOS as a selected
Platform
Helpshift uniquely identifies each registered App with a combination of 2 tokens:
Domain Name | This is your Helpshift domain name e.g. happyapps.helpshift.com |
Platform ID | Your App's unique App id |
You can find these by navigating to Settings > SDK (for Developers)
in your agent dashboard.
Select your App and check IOS as a platform from the dropdowns and copy
the 2 tokens to be passed when initializing Helpshift.
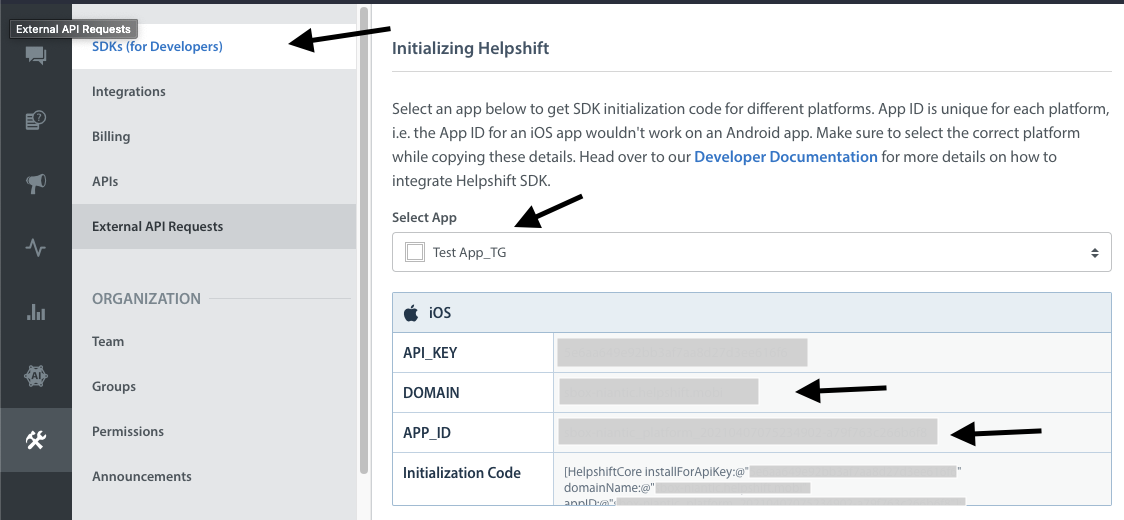
Initialize Helpshift by calling the method install(appId, domain) API
using Helpshift;
public class MyGameControl : MonoBehaviour
{
private HelpshiftSdk help;
...
void Awake() {
help = HelpshiftSdk.GetInstance();
var configMap = new Dictionary<string, object>();
help.Install(appId, domainName, configMap);
}
...
}
Initializing Helpshift in your app for China
A special install config key, isForChina
, should be used for integrating Helphift SDK for China region.
This config key accepts a boolean value. Pass true
if you are integrating the SDK in an app for China region. The value defaults to false
if left unspecified.
Initialize Helpshift by passing this key with appropriate value -
using Helpshift;
public class MyGameControl : MonoBehaviour
{
private HelpshiftSdk help;
...
void Awake() {
help = HelpshiftSdk.GetInstance();
var configMap = new Dictionary<string, object>()
{
{ "isForChina", true },
};
help.Install(appId, domainName, configMap);
}
...
}
Start using Helpshift
Helpshift is now integrated in your app. You should now use the support APIs inside your app to experience the functionality provided by the Helpshift SDK.