Outbound Support
With outbound support, you can proactively engage with consumers to solve problems within the app. Read more about the feature here.
All the public APIs in the SDK should be called after initializing the SDK via Helpshift.install() API
The steps to use this feature are the following -
To generate the link for outbound support, on your Helpshift dashboard, go to Settings > Workflows > Outbound Support.
You should see a New link button. Click on the New link button and select an action like Chat, Help Center, Single FAQ or FAQ Section and other data like CIFs, Tags, First User Message you want to send as payload to Helpshift SDK.
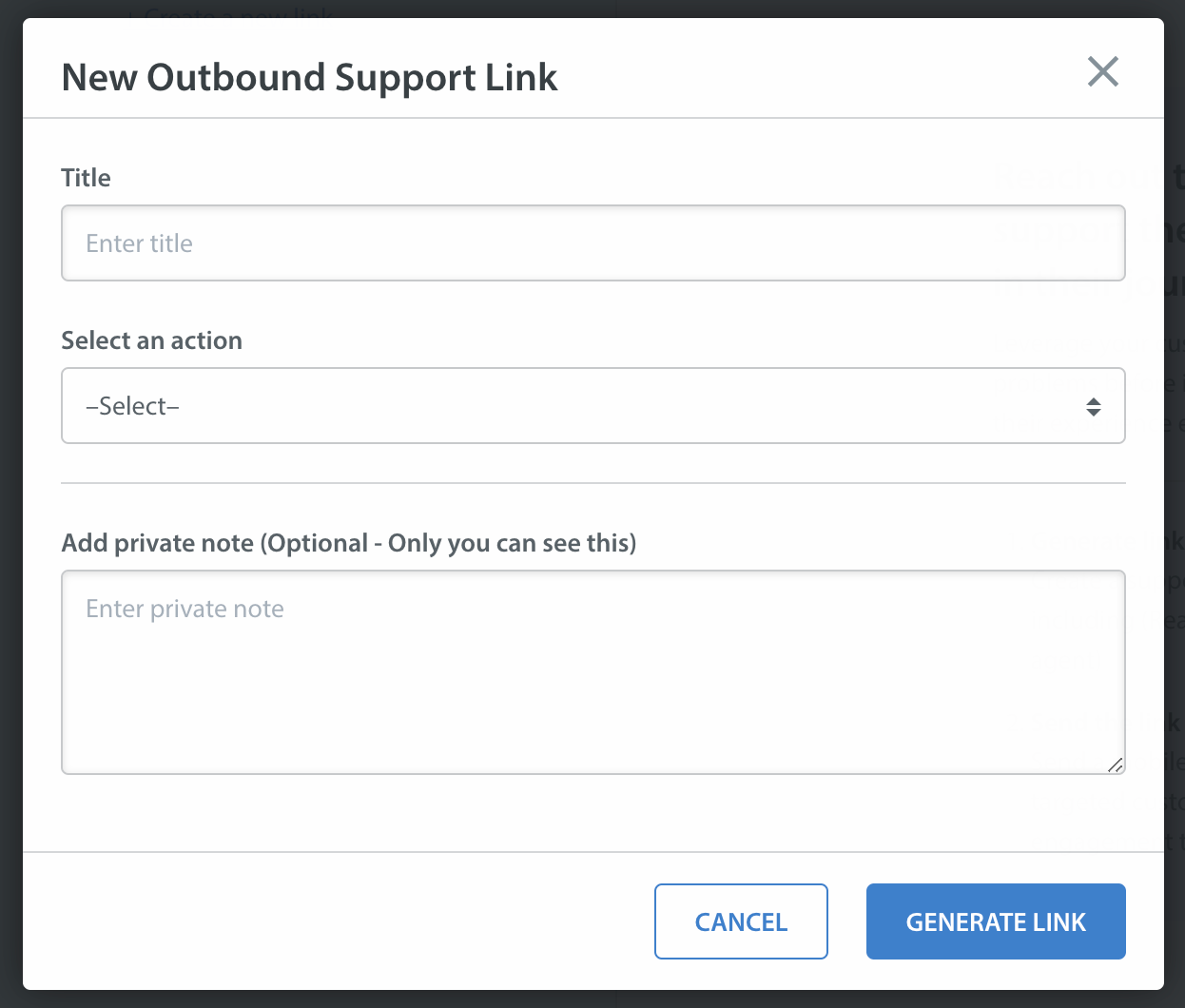
At last, you will get a URL encoded payload link. Send this link to your end-users embedded in a notification payload using your existing Push notification system.
YOUR_APP_IDENTIFIER: Can be any unique string that identifies your app. For example, like the scheme you would use in deep link URLs for your app like myApp , myAppSupport, etc.
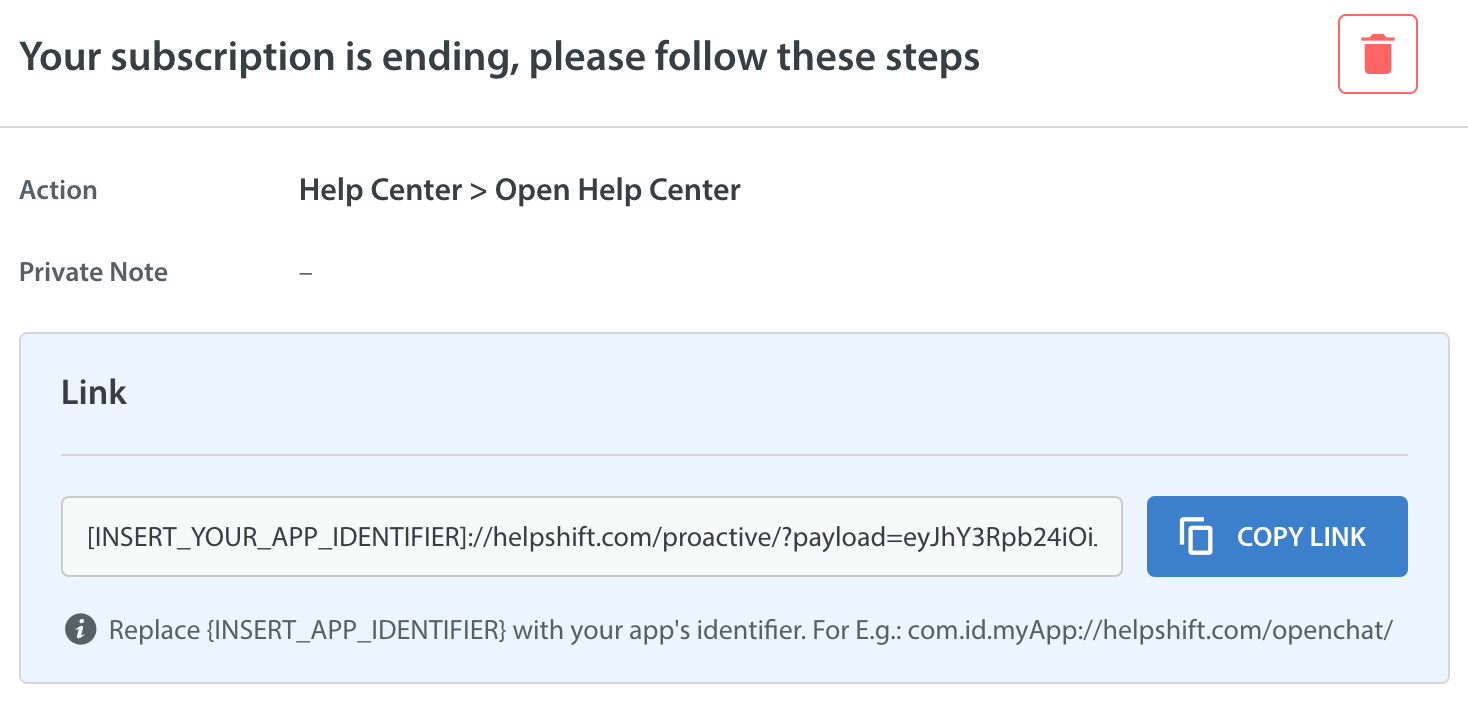
Delegate push notification data to Helpshift SDK
To pass the outbound support data to Helpshift, follow these steps -
Send push notification to the users you want to give outbound support using your app's existing push notification system
In your app, handle this notification such that when a user opens the app through notification, you pass the outbound support link in your notification data to Helpshift SDK by calling
handleProactiveLink(link)
.We will read the data from the link you provided and open Helpshift support with the configurations you provided from outbound support dashboard.
For example, following code shows how to handle incoming push notification and posting it on the notification bar of the device -
- Objective-C
- Swift
#import <HelpshiftX/Helpshift.h>
...
- (void) userNotificationCenter:(UNUserNotificationCenter *)center
willPresentNotification:(UNNotification *)notification
withCompletionHandler:(void (^)(UNNotificationPresentationOptions))completionHandler {
if([[notification.request.content.userInfo objectForKey:@"origin"] isEqualToString:@"helpshift"]) {
// This is a notification from Helpshift push notification system
[Helpshift handleNotificationWithUserInfoDictionary:notification.request.content.userInfo isAppLaunch:NO withController:self.window.rootViewController];
completionHandler(UNNotificationPresentationOptionNone);
} else {
// Present Notification Alert
completionHandler(UNNotificationPresentationOptionAlert);
}
}
import HelpshiftX
...
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
if notification.request.content.userInfo["origin"] as? String == "helpshift" {
Helpshift.handleNotification(withUserInfoDictionary: notification.request.content.userInfo, isAppLaunch: false, with: rootViewController)
completionHandler([])
} else {
// Handling for non-helpshift push notifications received when app is in foreground
completionHandler( [.alert])
}
}
You need to manage notifications for both Outbound Support and Helpshift notifications. As an example, on receiving Helpshift chat message notification, you can call
[Helpshift handleNotificationWithUserInfoDictionary:]
followed bycompletionHandler(UNNotificationPresentationOptionNone);
. On receiving Outbound Support notification, you can docompletionHandler(UNNotificationPresentationOptionAlert);
.This is just an example for reference. Actual implementation will depend on your app’s notification handling code.
When the user clicks on the notification, didReceiveNotificationResponse
delegate is called.
To delegate push notification data to Helpshift SDK, call [Helpshift handleProactiveLink:proactiveLink]
.
- Objective-C
- Swift
#import <HelpshiftX/Helpshift.h>
...
- (void) userNotificationCenter:(UNUserNotificationCenter *)center
didReceiveNotificationResponse:(UNNotificationResponse *)response
withCompletionHandler:(void (^)(void))completionHandler {
if([[response.notification.request.content.userInfo objectForKey:@"origin"] isEqualToString:@"helpshift"]) {
// This is a notification from helpshift push notification system
// for messages sent from agent from Helpshift Dashboard
[Helpshift handleNotificationWithUserInfoDictionary:response.notification.request.content.userInfo
isAppLaunch:YES
withController:self.viewController];
} else {
NSString* proactiveLink = [response.notification.request.content.userInfo objectForKey:@"helpshift_proactive_link"];
if (proactiveLink != nil) {
// This is your app's push notification system containing a Helpshift proactive link
[Helpshift handleProactiveLink:proactiveLink];
} else {
// This is your app's push notification system.
}
}
completionHandler();
}
import HelpshiftX
...
func userNotificationCenter(_ center: UNUserNotificationCenter,
didReceive response: UNNotificationResponse,
withCompletionHandler completionHandler: @escaping () -> Void) {
if let origin = response.notification.request.content.userInfo["origin"] as? String, origin == "helpshift" {
// This is a notification from helpshift push notification system
// for messages sent from agent from Helpshift Dashboard
Helpshift.handleNotification(withUserInfoDictionary: response.notification.request.content.userInfo,
isAppLaunch: true,
withController: self.viewController)
} else {
if let proactiveLink = response.notification.request.content.userInfo["helpshift_proactive_link"] as? String {
// This is your app's push notification system containing a Helpshift proactive link
Helpshift.handleProactiveLink(proactiveLink)
} else {
// This is your app's push notification system.
}
}
completionHandler()
}
Passing configuration specific to the current user
You may want to add configuration specific to the current user in your app when they click on the notification.
Setting local API config enables the Helpshift SDK to merge configuration from both, the config embedded in the outbound support link (as mentioned in previous steps) and the local config provided at runtime. This local API config is exactly same as we would expect in other APIs like showConversation:
or showFAQs:
.
We will use this configuration for current issue as well as next issue filed in same session.
You need to call this API after Helpshift Installation API and before Helpshift.handleProactiveLink()
Implement public interface HelpshiftProactiveAPIConfigCollectorDelegate
in your AppDelegate class and call setProactiveAPIConfigCollectorDelegate:
method to initialise the config collector delegate after call of install Helpshift SDK
You will have to implement the method getAPIConfig
where you can add any user specific config in the same format as you add in other public APIs like showConversation:
or showFAQs:
.
We will merge this config and the config embedded in the outbound support link. We will append data of config from outbound support link to local config like Tags, CIFs, etc. In case of conflicts, outbound support config will get the precedence.
For example, following code shows how to implement HelpshiftProactiveAPIConfigCollectorDelegate
and how to add user specific configurations using getAPIConfig
method -
- Objective-C
- Swift
// Set proactiveConfig collector delegate
[Helpshift.sharedInstance setProactiveAPIConfigCollectorDelegate:self];
// Delegate callback
- (nonnull NSDictionary *) getAPIConfig {
NSDictionary *config = @{ @"tags":tagsArray,
@"cifs": cifDictionary };
..
return localConfig;
}
// Set proactiveConfig collector delegate
Helpshift.sharedInstance.setProactiveAPIConfigCollectorDelegate(self)
// Delegate callback
func getAPIConfig() -> Dictionary<String, Any> {
var config: [String: Any] = [
"tags": tagsArray,
"cifs": cifDictionary
]
...
return config
}