Getting Started
Getting Started
Or, follow these simple steps to add Helpshift in-app support to your Android app right away -
Integration Guide
Version Requirements
Helpshift SDK Version | minSDKVersion | compileSDKVersion | buildToolsVersion |
---|---|---|---|
>= 7.9.0 | 21 | 30 | 30 |
>= 7.6.0 | 21 | 29 | 29 |
>= 7.3.0 | 21 | 28 | 28 |
>= 6.2.0 | 21 | 26 | 26 |
For example, update your AndroidManifest.xml
as follows:
<uses-sdk android:minSdkVersion="14" android:targetSdkVersion="28"
android:compileSDKVersion="28" android:buildToolsVersion="28" />
- If you have released your app with
7.8.0
version, we highly recommend that you upgrade to7.8.1
which fixes an important migration bug. Refer here. - If you're currently using Android SDK
v7.7.0
orv7.7.1
in your app, please update the SDK tov7.7.2
which contains some important bug fixes. Check release notes here. - If you have released your app with Android SDK
v7.7.0
andv7.7.1
to production, please get in touch with us via support and make sure to upgrade tov7.7.2
asap. Apologies for any inconvenience that this has caused.
Download the SDK
Gradle based projects
Add the following dependencies to your build.gradle
file inside the depencencies section.
dependencies {
implementation 'com.android.support:design:28.0.0'
implementation 'com.android.support:recyclerview-v7:28.0.0'
implementation 'com.android.support:cardview-v7:28.0.0'
implementation 'com.helpshift:android-helpshift-aar:v7.11.2'
}
design
, cardview
and recyclerview
library are required by the Helpshift SDK, make sure they've also been added.
Helpshift SDK comes with built-in support for 47 languages. If your app supports only a subset of these languages, please follow the steps mentioned here.
Helpshift is now ready to help you have conversations with your users!
Move on to Initializing Helpshift in your App.
IntelliJ/Eclipse based projects
If your Android project is based on IntelliJ or Eclipse, download the latest version of the Android SDK.
The zip file contains the Helpshift SDK in the Android Library Project format.
- IntelliJ Integration: follow the steps here.
- Eclipse Integration: follow the steps here.
Maven based projects
If your Android project is based on Maven, follow the steps here.
Start using Helpshift
Helpshift is now integrated in your app and ready to provide customer support and collect meaningful data about your users.
Initialize Helpshift in your App
Helpshift uniquely identifies each registered App with a combination of 3 tokens:
API Key | Your unique developer API key |
Domain Name | Your Helpshift domain name without any http: or slashes. E.g. happyapps if your account is happyapps.helpshift.com |
App ID | Your App's unique ID |
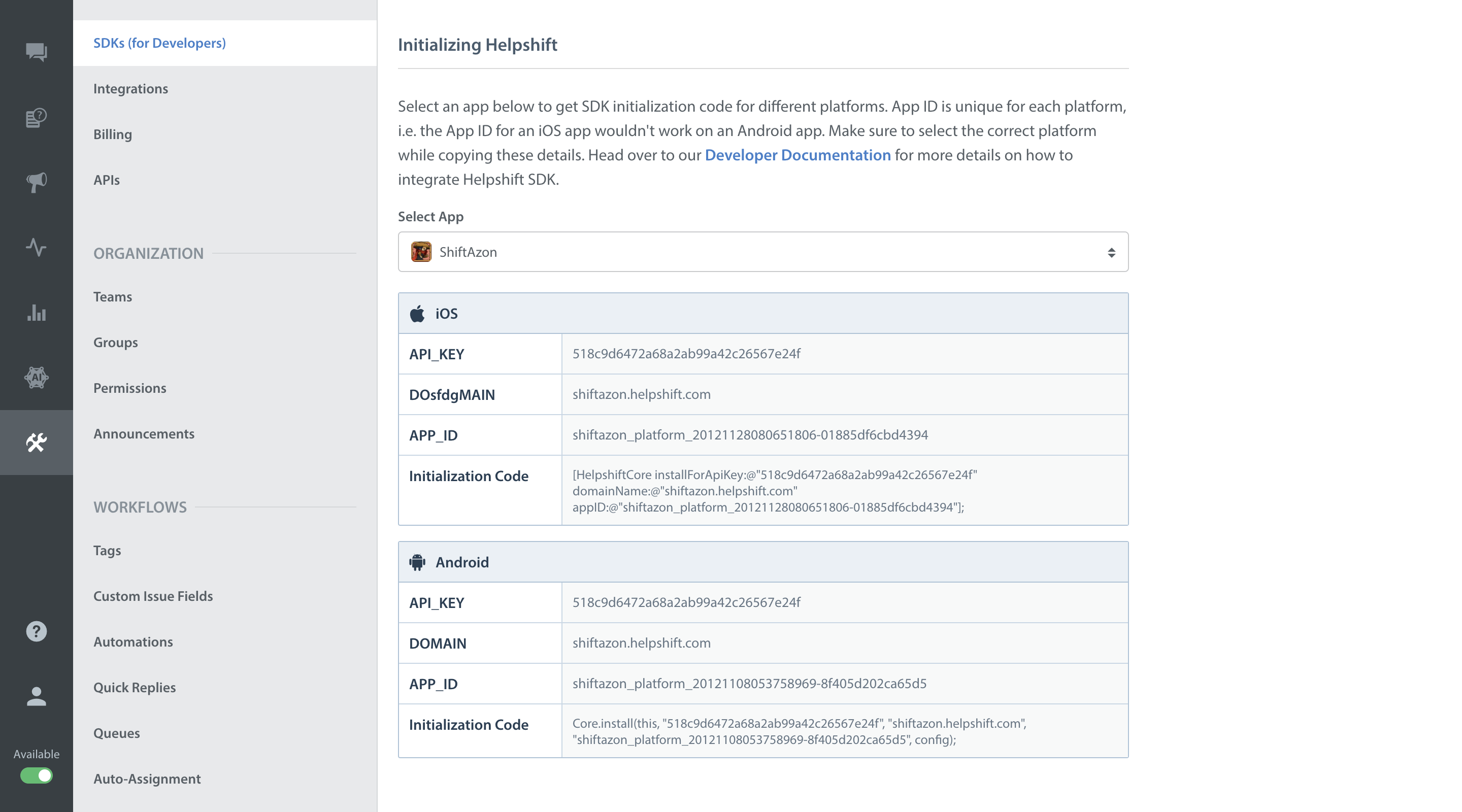
Initialize Helpshift by calling the com.helpshift.Core
's install
method by doing the following.
- Initialize the
Core
class with the Helpshift service that you want to use. - Call the
Core
'sinstall
method.
com.helpshift.Core
is the class which encapsulates all the functions which are common to all Helpshift services.
- Using InstallConfig
- Using Map
import com.helpshift.Core;
import com.helpshift.support.Support;
import com.helpshift.InstallConfig;
import com.helpshift.exceptions.InstallException;
...
...
InstallConfig installConfig = new InstallConfig.Builder().build();
...
Core.init(Support.getInstance());
try {
Core.install(this,
"YOUR_API_KEY",
"YOUR_DOMAIN_NAME",
"YOUR_APP_ID",
installConfig);
} catch (InstallException e) {
Log.e(TAG, "invalid install credentials : ", e);
}
import com.helpshift.Core;
import com.helpshift.support.Support;
...
...
HashMap config = new HashMap();
...
Core.init(Support.getInstance());
try {
Core.install(this,
"YOUR_API_KEY",
"YOUR_DOMAIN_NAME",
"YOUR_APP_ID",
config);
} catch (InstallException e) {
Log.e(TAG, "invalid install credentials : ", e);
}
Placing the install call
You should not place the install call anywhere other than Application.onCreate
Placing it elsewhere might cause unexpected runtime problems.
HelpshiftInitializationException
From v7.4.0 & above, calling any API before the install call would throw an unchecked HelpshiftInitializationException in debug mode.
InstallException
From v4.6.0 & above, the Helpshift install call will throw InstallException in case the validation of the SDK keys fail.
Multi-process apps
If your app is a multi-process app, please visit the troubleshooting guide here to integrate the Helpshift SDK with your app.
Minimum supported Android version
From SDK version 4.4.0 onwards, the Helpshift Android SDK requires minimum API level to be 14. For versions 14 and 15 you will not receive the rich push notifications which are only supported after android api level 16.
With the transition to TLSv1.2 on Sep 20,2021, connections to a Helpshift SDK via any previous TLS version will not work as expected. Specifically, this means that:
- Helpshift SDKs will not work or load on lower-end Android devices (meaning, Android 4.x and earlier), because these lower-end Android versions do not support TLSv1.2.
- End-users on lower-end Android devices will not be able to access FAQs, raise new issues, or access their past support conversations.
- On a lower-end Android device, the mobile app that embeds a Helpshift SDK will be prevented from communicating with the SDK for any purpose. However, this disconnect will not cause any malfunction or crash on the parent application.
Permissions needed by Helpshift SDK
The Helpshift Android SDK requires WRITE_EXTERNAL_PERMISSION
. For SDK v6.2.0 and above this permission is optional, and you can choose to not include this permission in your app’s manifest file. The behaviour of the SDK based on whether this permission is mentioned in your manifest file is detailed here.
Troubleshooting
If you are having issues with Helpshift integration, head over to the Troubleshooting section for further information.
Additional Configuration
- If you are using Proguard, you will need to add the following to your project's
proguard-project.txt
file.
# For Helpshift SDK <= 4.8.1
-keepnames class * extends com.helpshift.support.fragments.MainFragment
# For Serializable classes
-keepnames class * implements java.io.Serializable
-keepclassmembers class * implements java.io.Serializable {
static final long serialVersionUID;
private static final java.io.ObjectStreamField[] serialPersistentFields;
!static !transient <fields>;
private void writeObject(java.io.ObjectOutputStream);
private void readObject(java.io.ObjectInputStream);
java.lang.Object writeReplace();
java.lang.Object readResolve();
}
# If the app uses support libs version 23 or below
-keepclassmembernames class android.support.v4.app.Fragment {
android.support.v4.app.FragmentManagerImpl mChildFragmentManager;
}
# Support design
-dontwarn android.support.design.**
-keep class android.support.design.** { *; }
-keep interface android.support.design.** { *; }
-keep public class android.support.design.R$* { *; }
# Appcompat
-keep public class android.support.v7.widget.** { *; }
-keep public class android.support.v7.internal.widget.** { *; }
-keep public class android.support.v7.internal.view.menu.** { *; }
-keep public class * extends android.support.v4.view.ActionProvider {
public <init>(android.content.Context);
}
# Cardview
# Based on this issue http://stackoverflow.com/questions/29679177/cardview-shadow-not-appearing-in-lollipop-after-obfuscate-with-proguard/29698051
-keep class android.support.v7.widget.RoundRectDrawable { *; }
Proguard rules for Android support libraries are taken from the `android-proguard-snippets` repository, which you can review [here](https://github.com/krschultz/android-proguard-snippets)
Helpshift Android SDK **v2.8.2 and above** is not pre-obfuscated with Proguard. To shrink & obfuscate the Helpshift SDK, we recommend you use this Proguard configuration in your project.
/.notes/
Proguard is recommended for shrinking, optimizing, and
obfuscating your code by removing unused code and renaming classes,
fields, and methods with semantically obscured names. The result is
a smaller sized APK file that is more difficult to reverse
engineer. For more information refer
[Proguard Documentation](http://developer.android.com/tools/help/proguard.html)
In your
build.xml
file, insert the following between the<target name="-post-compile">
tags. This will check the Helpshift xml files for the requisite resources and warn you if any of those resources are missing. If the "-post-compile" target is missing, wrap the below code snippet with<target name="-post-compile">...</target>
tags.<path id="helpshift-classpath">
<fileset dir="<path/to/Helpshift-SDK/libs>">
<include name="Helpshift.jar"/>
</fileset>
</path>
<java classname="com.helpshift.HSPostCompileCheck" failonerror="true">
<classpath refid="helpshift-classpath"/>
<arg value="<YOUR_APPLICATION_NAMESPACE>"/>
</java>