Getting Started Android
You're 3 steps away from adding great in-app support to your Unity game.
Guide to integrating the Unity plugin for the Helpshift SDK X which you can call from your C# and Javascript game scripts.
Requirements
Unity 5.5.6 and above.
SDK X is functional only above API Level 24 i.e Android OS 7 Nougat and above.
The
minSDKVersion
in code is kept as API Level 16 to allow importing the SDK and compiling in apps that allow installation till API Level 16 i.e Android 4.4 Jelly Bean.If your app is installed on a device below API level 24 (i.e below Android OS 7 Nougat) then you can choose to have a different medium for providing support. For example, Email, Web etc.
- Update (August 2023): SDK X 10.2.x is functional only above API Level 24 i.e Android OS 7 Nougat and above.
- SDK X 10.3.0 is supported on Android 7 and above (API level 24 and above). You can still compile your app using a minimum API level of 16, but the support starts for API level 24 and above. For details, please refer to this article.
Download Helpshift Unity SDK
1. SDK X - In-app Customer Service
Helpshift SDK .zip folder includes:
helpshiftX-plugin-unity-v10.3.1.unitypackage | Unity package of Helpshift SDK X |
unity-jar-resolver (v1.2.170.0) | Resolves Android Helpshift package support lib dependencies. |
Add Helpshift to your Unity project
- Unzip the Helpshift Unity SDK package.
- Helpshift Unity SDK appears as a standard
.unitypackage
which you can import through the Unity package import procedure. - Following are the steps to import the
helpshiftx-plugin-unity-version.unitypackage
into your Unity game:- In the Open Unity project, navigate to Assets drop-down menu and select the Import Package > Custom Package
- From the unzipped SDK, select
helpshiftx-plugin-unity-version.unitypackage
file to import the Helpshift SDK. - In the Import Unity Package window, click Import
- If you are also integrating for iOS, select
iOS/Helpshift.framework
folder while importing the unitypackage. Refer here.
Resolve Android Appcompat library requirements
Helpshift SDK depends on android appcompat libraries. You can get these libraries in one of the following ways depending on the build process that you use.
Resolve dependency when using Unity's Internal or Unity's internal Gradle build system
Use Unity Jar Resolver plugin to download and integrate android library dependencies.
If your project already uses the Unity Jar Resolver, you can skip the Unity Jar Resolver importing step.
You can use any version of Unity Jar Resolver as per your needs. Helpshift packages this for convenience of developers.
You can find the Unity Jar Resolver releases here: https://github.com/googlesamples/unity-jar-resolver/tags
Import the Unity Jar Resolver plugin into your Unity project
- In the Open Unity project, navigate to Assets drop-down menu and select the Import Package > Custom Package
- From the unzipped Helpshift SDK, select
unity-jar-resolver/external-dependency-manager-1.2.170.unitypackage
file to import the Unity Jar resolver. - In the Import Unity Package window, click Import
If the Unity Jar resolver wants to enable Android Auto-resolution, click the Enable button, to resolve all the dependencies automatically on changing any dependency file. You can enable or disable the settings using Assets > Play Services Resolver > Android Resolver > Settings
By default, the Unity jar resolver auto-resolves all the required dependencies. Following are the steps to resolve dependencies manually:
- In the Open Unity project, navigate to Assets dropdown menu and choose Play services resolver > Android Resolve
- Select the Resolve or Force Resolve
To know more about the Unity Jar Resolver, refer to: Unity Jar Resolver
Resolve dependency when using custom Gradle template or Export Project
Unity's in-built gradle build support and exporting to android studio does not support per plugin gradle script. Therefore, by default, Helpshift SDK cannot add the dependencies by itself.
The mainTemplate.gradle
is genereted when you enable the Custom Gradle Template property on the Player window.
The build.gradle
exists in generated Gradle project when you enable the Export Project property on the Player window and Build the project.
Update dependencies section of the mainTemplate.gradle
or build.gradle
file as:
- 10.3.X
- Below 10.2.3
dependencies {
implementation fileTree(dir: 'libs', include: ['*.jar'])
implementation(name: 'Helpshift', ext:'aar')
implementation 'androidx.appcompat:appcompat:1.0.0'
}
dependencies {
implementation fileTree(dir: 'libs', include: ['*.jar'])
implementation(name: 'Helpshift', ext:'aar')
implementation 'com.android.support:appcompat-v7:28.0.0'
}
Please refer to Unity's Gradle template documentation here: https://docs.unity3d.com/Manual/gradle-templates.html
Initializing Helpshift in your app
To use Helpshift's APIs, please import the Helpshift's namespace like below
using Helpshift;
First, create an app on the Helpshift Dashboard
Create an app with Android as a selected
Platform
Helpshift uniquely identifies each registered App with a combination of 2 tokens:
Domain Name | Your Helpshift domain. E.g. happyapps.helpshift.com |
Platform ID | Your App's unique App Id |
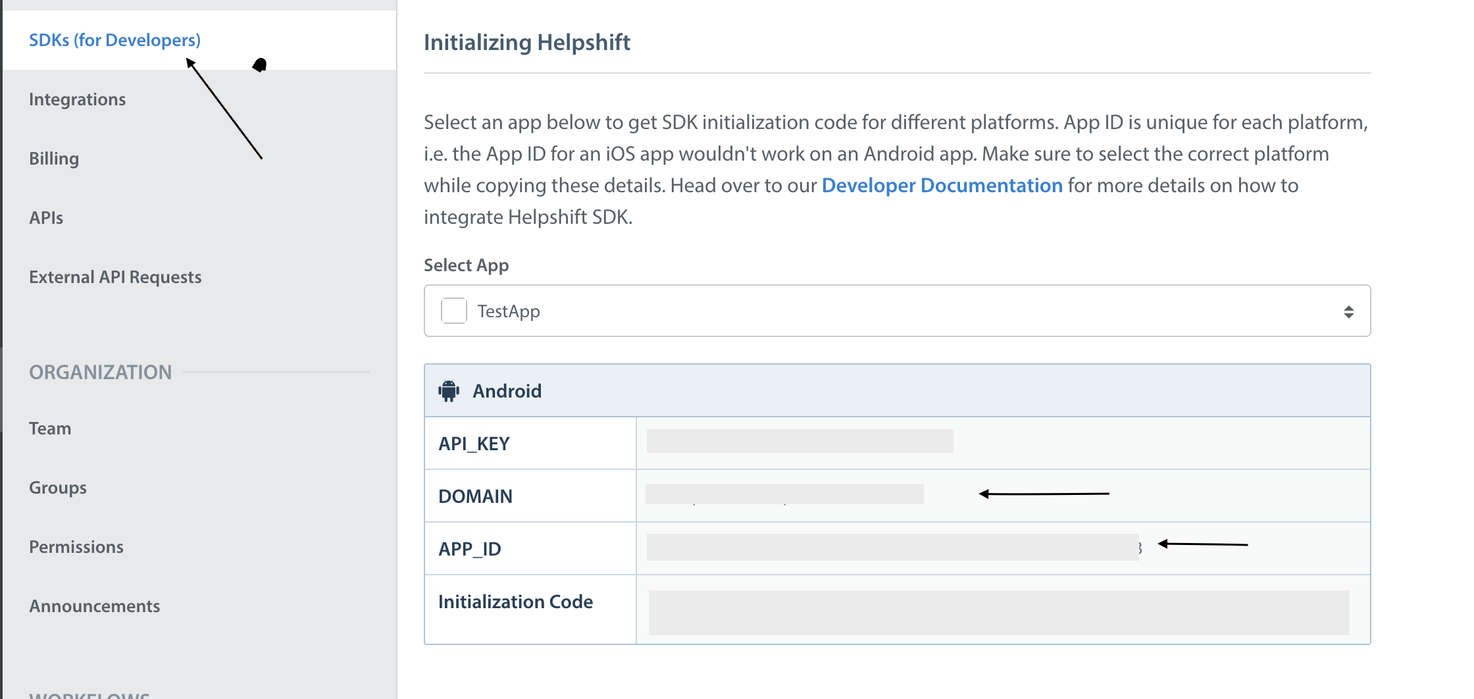
Initialize Helpshift by calling the method install(appId, domain) API
using Helpshift;
public class MyGameControl : MonoBehaviour
{
private HelpshiftSdk help;
...
void Awake() {
help = HelpshiftSdk.GetInstance();
var configMap = new Dictionary<string, object>();
help.Install(appId, domainName, configMap);
}
...
}
Initializing Helpshift in your app for China
A special install config key, isForChina
, should be used for integrating Helphift SDK for China region.
This config key accepts a boolean value. Pass true
if you are integrating the SDK in an app for China region. The value defaults to false
if left unspecified.
using Helpshift;
public class MyGameControl : MonoBehaviour
{
private HelpshiftSdk help;
...
void Awake() {
help = HelpshiftSdk.GetInstance();
var configMap = new Dictionary<string, object>();
configMap.Add("isForChina", true);
help.Install(appId, domainName, configMap);
}
...
}
Placing the install call
You should place install call inside the Awake()
method. Placing it elsewhere might cause unexpected runtime problems.
HelpshiftInitializationException
Calling any API before the install call would throw an unchecked HelpshiftInitializationException in debug mode.
Android OS version Support
Calling install()
below android SDK version 24 will not work. All the APIs will be non operable.
Start using Helpshift
Helpshift is now integrated in your app. You should now use the support APIs for conversation screens inside your app.
Run your app, and try starting a test conversation using the ShowConversation API call. Then goto your Helpshift agent dashboard and reply to experience the in-app messaging.
Sample usage for FAQs and conversation APIs:
public class MyGameControl : MonoBehaviour
{
private HelpshiftSdk help;
void Awake(){
// install call here
}
void OnGUI () {
...
var configMap = new Dictionary<string, object>();
// Starting a conversation with your customers
if (MenuButton (contactButton))
{
help.ShowConversation(configMap);
}
}
}