Getting Started
Follow these simple steps to add Helpshift in-app support to your Android app right away.
Requirements
If you are on SDK X or migrating to SDK X please note the following requirements:
- SDK X is functional only above API Level 24 i.e Android OS 7 Nougat and above.
- The
minSDKVersion
in code is kept as API Level 19 to allow importing the SDK and compiling in apps that allow installation till API Level 19 i.e Android 4.4 KitKat. - If your app is installed on a device below API level 24 (i.e below Android OS 7 Nougat) then you can choose to have a different medium for providing support. For example, Email, Web etc. We throw an
UnsupportedOSVersionException
if the SDK is initialized below API Level 24. Please catch and use this exception according to your needs. Refer: Initializing the SDK.
- From 10.4.0, SDK is compiled using Java 8 to ensure compatibility with Android 15 build tool chain.
- SDK 10.3.0 and above functions and is supported on Android 7 and above (API level 24 and above).
- You can still compile your app using a minimum API level of 19, but the support starts for API level 24 and above. For details regarding Helpshift SDK's Android OS support policy, please refer to this article.
Gradle based projects
Add the following dependencies to your build.gradle
file inside the dependencies section.
- Above 10.3.X
- Below 10.2.2
dependencies {
// androidx support library dependency
implementation 'androidx.appcompat:appcompat:1.0.0'
implementation 'com.helpshift:helpshift-sdkx:10.4.0'
}
dependencies {
// legacy android support library dependency
implementation 'com.android.support:appcompat-v7:28.0.0'
implementation 'com.helpshift:helpshift-sdkx:10.2.X'
}
Helpshift SDK comes with built-in support for 47 languages. An API to change SDK language is provided, you can check here.
Helpshift is now ready to help you have conversations with your users!
Start using Helpshift
Version Requirements
Helpshift SDK Version | minSDKVersion | compileSDKVersion | buildToolsVersion |
---|---|---|---|
>= 10.4.0 | 19 | 35 | 35 |
>= 10.3.0 | 16 | 34 | 34 |
>= 10.2.0 | 16 | 33 | 33 |
>= 10.0.2 | 16 | 31 | 31 |
>= 10.0.0 | 16 | 29 | 29 |
Initialize Helpshift in your App
First, create an app on the Helpshift Dashboard
Create an app with Android as a selected
Platform
Helpshift uniquely identifies each registered App with a combination of 2 tokens:
Platform ID | Your App's unique platform id (App's App Id on dashboard is your platform Id) |
Domain Name | Your Helpshift domain. E.g. happyapps.helpshift.com |
To obtain these values:
- Go to your Helpshift dashboard
- Navigate to
Settings
>SDKs (for Developers)
- Select your App and from the dropdowns
- Copy the two tokens present in the "Android" table
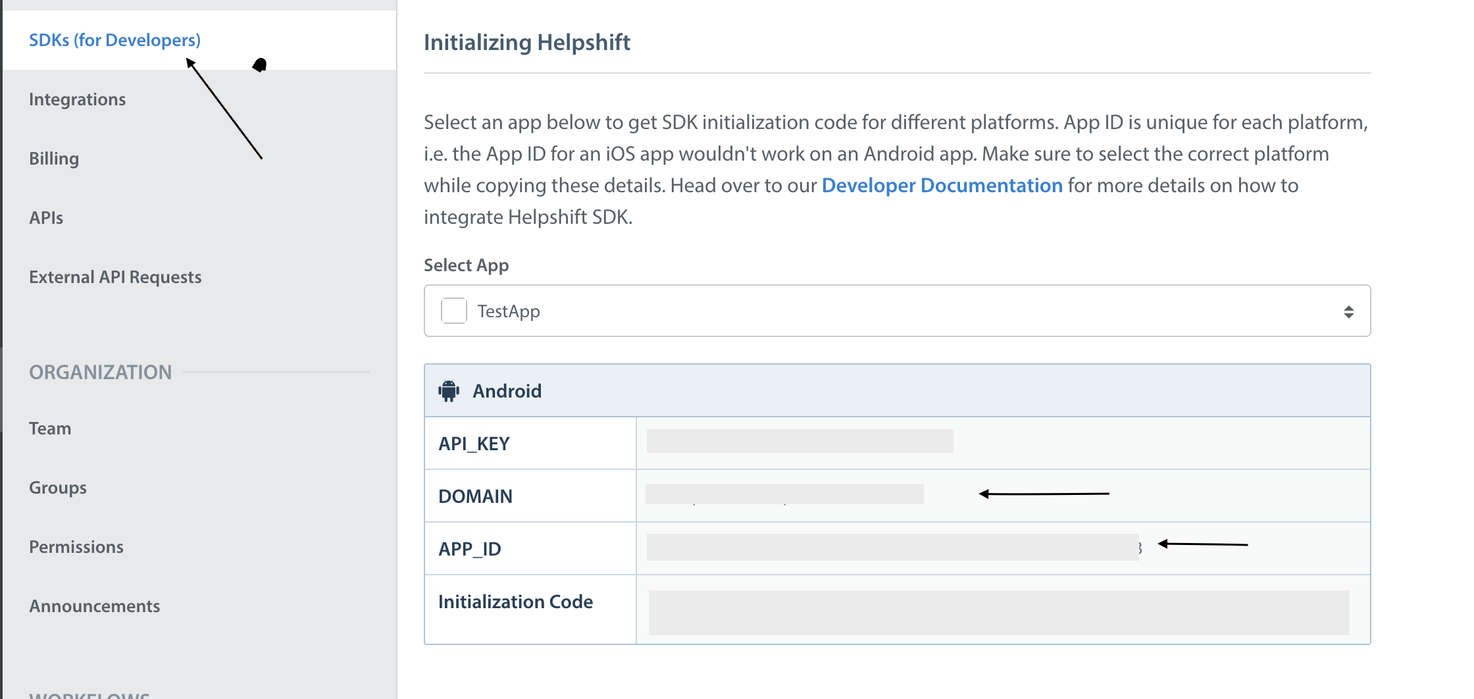
Initialize SDK by calling com.helpshift.Helpshift.install()
method.
You can optionally pass the configuration map to Helpshift.install()
API. To see supported configurations, check the SDK Configuration page.
public class MainApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
// Add install configs in the config map
Map<String, Object> config = new HashMap<>();
//...
// Install call
try {
Helpshift.install(this,
"<App Id from the Helpshift Dashboard>",
"<Domain name from the Helpshift Dashboard>",
config);
} catch (UnsupportedOSVersionException e) {
// Android OS versions prior to Android 7 Nougat (< API Level 24) are not supported.
// SDK will be non-operational so you can choose to provide support to your users via other mediums like Web, Email etc.
}
}
}
Initializing Helpshift in your app for China
A special install config key, isForChina
, should be used for integrating Helphift SDK for China region.
This config key accepts a boolean value. Pass true
if you are integrating the SDK in an app for China region. The value defaults to false
if left unspecified.
public class MainApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
// Add install configs in the config map
Map<String, Object> config = new HashMap<>();
config.put("isForChina", true);
//...
// Install call
try {
Helpshift.install(this,
"<App Id from the Helpshift Dashboard>",
"<Domain name from the Helpshift Dashboard>",
config);
} catch (UnsupportedOSVersionException e) {
// Android OS versions prior to Android 7 Nougat (< API Level 24) are not supported.
// SDK will be non-operational so you can choose to provide support to your users via other mediums like Web, Email etc.
}
}
}
Placing the install call
You should not place the install call anywhere other than Application.onCreate()
. Placing it elsewhere might cause unexpected runtime problems.
HelpshiftInitializationException
Calling any API before the install call would throw an unchecked HelpshiftInitializationException
in debug mode.
UnsupportedOSVersionException
Calling install()
below Android SDK version 24 will throw this checked exception. All the APIs will be non operable.