Notifications iOS
Notifications iOS
Configure Push and In-App notifications.
Configure push notifications via Helpshift
Notifications can be sent to your users when you reply to an issue submitted by them. In addition to the expected Push Notification behavior on iOS, you can customize your notifications to display a numbered badge on your App icon or play a sound alert when a notification is received.
If your app does not already use push, you will need to enable push for your app. To enable push notification in your application you need to add APNS registration code to your AppDelegate's application:didFinishLaunchingWithOptions:
method:
- Using UILocalNotification framework
- Using UNUserNotification framework
- (BOOL) application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
...
UIUserNotificationType notificationType = UIUserNotificationTypeBadge |UIUserNotificationTypeAlert;
UIUserNotificationSettings *notificationSettings =[UIUserNotificationSettings settingsForTypes:notificationType categories:nil;
[[UIApplication sharedApplication]registerUserNotificationSettings:notificationSettings];
[[UIApplication sharedApplication] registerForRemoteNotifications];
...
}
- (BOOL) application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
...
UNUserNotificationCenter *center = [UNUserNotificationCenter currentNotificationCenter];
center.delegate = self;
[center requestAuthorizationWithOptions:(UNAuthorizationOptionBadge | UNAuthorizationOptionSound | UNAuthorizationOptionAlert)
completionHandler:^(BOOL granted, NSError *_Nullable error) {
...
}];
[UIApplication sharedApplication registerForRemoteNotifications];
...
}
Configure Helpshift's push notification service in the Helpshift admin interface
You can configure this in 4 simple steps:
Setup your application with Apple and enable Push notifications.
You need to generate certificates for APNS on Apple's portal that you can later provide to Helpshift. This lets Helpshift send push notifications to your users. Apple has moved away from old type of certificates to Apple Push Notification service SSL. There is another option to create Apple Push Notification Authentication Key, but Helpshift does not support this option yet. Its also possible that you still have the old type of certificate that has not yet expired, this is also supported by Helpshift.
Apple provides two variations of Apple Push Notification service SSL
- Apple Push Notification service SSL (Sandbox)
- Apple Push Notification service SSL (Sandbox & Production)
After creating your certificate, download it. Double click on it to import it to the Keychain Access Application. In the Keychain Access Application, right click on the certificate that was just added, and click export it in .p12 format. Please provide a password while exporting the certificate since we do not accept empty passwords. (Note that if your development private key is not present in Keychain Access, you will not be able to export it in .p12 format.) After export, login and upload the .p12 file in your app in the Helpshift admin panel. Provide the same password you used while exporting to .p12 format.
Please note that you need to use the same bundle identifier in the app as the one you used to create the APNS certificate.
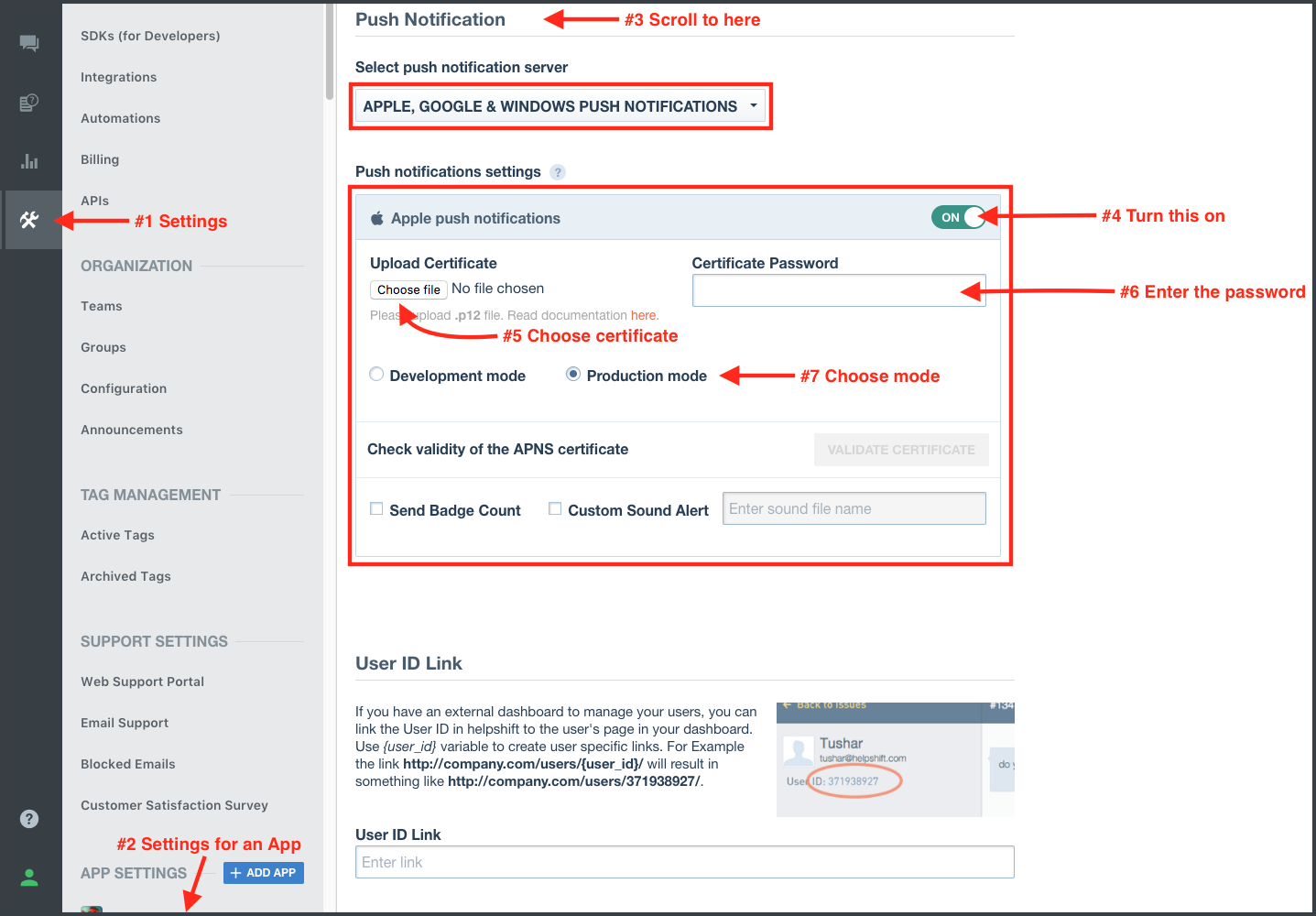
You can configure whether to send a badge or not, and sound alerts if you provided custom sounds bundled with your app to handle notifications. Save it and you're all set.
Development (Sandbox) mode vs. Production mode
When you build and run your app from Xcode, it is in development (Sandbox) mode. To test push notifications from Helpshift in this mode make sure you choose 'Development Mode' while uploading either of the above two certificate types.
When you publish your app and download from App Store, your app is in Production mode. To test push notifications from Helpshift in production mode make sure you choose 'Production Mode' while uploading a certificate of 'Apple Push Notification service SSL (Sandbox & Production)' type. Sandbox mode certificate will not work on a production environment.
We do not support using the same certificate for both sandbox and production apps. In these cases we recommend you create two separate apps on our dashboard, one in 'Production mode' and the other in 'Development mode'. While testing please use the credentials of the developement mode app. When you are ready to publish, please replace the credentials with those of the production level app.
Your Push Certificate has an expiry date, as indicated in the below screenshot. Helpshift will not send you a reminder when your Push Certificate expires, so please make sure that your developer keeps a tab on the expiry date to reupload the Push Certificate.
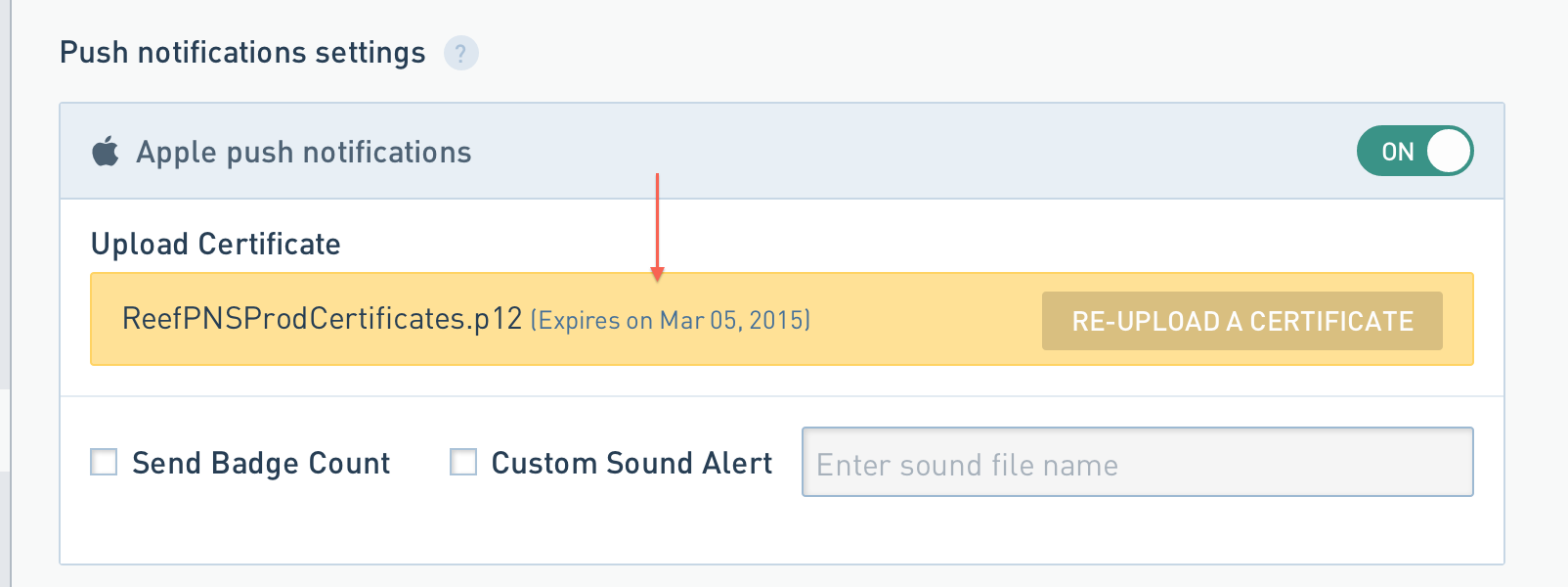
Configure the Helpshift iOS SDK to handle notifications
When push is not configured, Helpshift SDK shows out-of-the-box "in-app notifications" for every message sent by Agents/Bots.
You should call registerDeviceToken
API only after you have configured the Helpshift dashboard for push notifications. Calling the registerDeviceToken
API without configuring the Helpshift dashboard will stop showing out-of-the-box "in-app notifications" for the end users.
- Using UILocalNotification Framework
- Using UNUserNotification framework
For Helpshift SDK to work with the Helpshift push notification service you will need to invoke the `registerDeviceToken:` API call inside the application delegate method `application:didRegisterForRemoteNotificationsWithDeviceToken:`
- (void) application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken {
[HelpshiftCore registerDeviceToken:deviceToken];
}
When a push notification is received, the application delegate didReceiveRemoteNotification: is called. Additionally:
- If the application was not in the foreground, and the user taps the push notification, this method is called again.
- In cases where the app might have been killed by the user, and then they tap on a push notification, the application's didFinishLaunchingWithOptions: delegate is called.
In all of the above cases, developers should check the "origin" field of the notification dictionary and call handleRemoteNotification:isAppLaunch:withController: API if the origin of the notification is "Helpshift". The Helpshift SDK will check Issues for which the notifications were received and will launch the Conversation screen for those Issues automatically. The isAppLaunch boolean flag here is used to distinguish between an active or backgrounded app vs. an app that was killed by the user. In the latter case, this flag should be set to true.
Example usage:
When receiving remote notifications when the app starts for the first time, or after being killed by the user:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
app->run();
...
if(launchOptions[UIApplicationLaunchOptionsRemoteNotificationKey]) {
NSDictionary *userInfo = launchOptions[UIApplicationLaunchOptionsRemoteNotificationKey];
if([[userInfo objectForKey:@"origin"] isEqualToString:@"helpshift"]) {
[HelpshiftCore handleRemoteNotification:userInfo
isAppLaunch:true
withController:self.window.rootViewController];
}
} else if(launchOptions[UIApplicationLaunchOptionsLocalNotificationKey]) {
[self application:application didReceiveLocalNotification:launchOptions[UIApplicationLaunchOptionsLocalNotificationKey]];
}
...
}
For the didReceiveRemoteNotification delegate -
- (void) application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo {
if([[userInfo objectForKey:@"origin"] isEqualToString:@"helpshift"]) {
[HelpshiftCore handleRemoteNotification:userInfo
isAppLaunch:false
withController:self.window.rootViewController];
}
}
If you need to handle badge reset in the application icon, you can do
something like below in the applicationDidBecomeActive:
delegate
method:
- (void)applicationDidBecomeActive:(UIApplication *)application
{
[[UIApplication sharedApplication] setApplicationIconBadgeNumber:0];
}
- To test Push Notifications for an app that is already in production please refer to this.
- You should check the origin and call the
handleRemoteNotfication
as soon as you get the callback. SDK checks the state of the app (Active / Inactive) and based on it, decides to show chat screen or in-app notification. Delay in calling handleRemoteNotification could result in SDK to get inaccurate application state.
For Helpshift SDK to work with the Helpshift push notification service you will need to invoke the `registerDeviceToken:` API call inside the application delegate method `application:didRegisterForRemoteNotificationsWithDeviceToken:`I
- (void) application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken {
[HelpshiftCore registerDeviceToken:deviceToken];
}
To respond to the delivery of notifications, you must implement a delegate for the shared UNUserNotificationCenter object. Your delegate object must conform to the UNUserNotificationCenterDelegate protocol, which the notification center uses to deliver notification information to your app:
- If a notification arrives while your app is in the foreground, UNUserNotificationCenterDelegate's
willPresentNotification:
is called. - The system does not call the
userNotificationCenter:willPresentNotification:withCompletionHandler:
method when your app is in the background or is not running. In those cases, the system alerts the user according to the information in the notification itself. When the user selects an action from the notification interface, the system notifies your app of the user's choice. To receive responses, your delegate object must implement theuserNotificationCenter:didReceiveNotificationResponse:withCompletionHandler:
method.
In all of the above cases, you should check the "origin" field of the notification dictionary and call handleNotificationWithUserInfoDictionary:isAppLaunch:withController:
API if the origin of the notification is "helpshift". The Helpshift SDK will check Issues for which the notifications were received and will launch the Conversation screen for those Issues automatically. The isAppLaunch boolean flag here is used to distinguish between an active or backgrounded app vs. an app that was killed by the user. In the latter case, this flag should be set to true.
Example usage:
For the userNotificationCenter:willPresentNotification:withCompletionHandler: delegate -
- (void) userNotificationCenter:(UNUserNotificationCenter *)center
willPresentNotification:(UNNotification *)notification
withCompletionHandler:(void (^)(UNNotificationPresentationOptions options))completionHandler {
if([[notification.request.content.userInfo objectForKey:@"origin"] isEqualToString:@"helpshift"]) {
[HelpshiftCore handleNotificationWithUserInfoDictionary:notification.request.content.userInfo
isAppLaunch:false
withController:self.window.rootViewController];
}
completionHandler(...);
}
For the userNotificationCenter:didReceiveNotificationResponse:withCompletionHandler: delegate -
- (void) userNotificationCenter:(UNUserNotificationCenter *)center didReceiveNotificationResponse:(UNNotificationResponse *)response withCompletionHandler:(void (^)(void))completionHandler {
if([[response.notification.request.content.userInfo objectForKey:@"origin"] isEqualToString:@"helpshift"]) {
[HelpshiftCore handleNotificationResponseWithActionIdentifier:response.actionIdentifier
userInfo:response.notification.request.content.userInfo
completionHandler:completionHandler];
}
completionHandler();
}
If you need to handle badge reset in the application icon, you can do
something like below in the applicationDidBecomeActive:
delegate
method:
- (void)applicationDidBecomeActive:(UIApplication *)application
{
[[UIApplication sharedApplication] setApplicationIconBadgeNumber:0];
}
- To test Push Notifications for an app that is already in production please refer to this.
- You should check the origin and call the
handleNotificationWithUserInfoDictionary
as soon as you get the callback. SDK checks the state of the app (Active / Inactive) and based on it, decides to show chat screen or in-app notification. Delay in calling handleRemoteNotification could result in SDK to get inaccurate application state.
In-app notifications
In-app notifications are similar to Apple's push notification banners. Unlike push notifications, they appear within your app when it is in use by the user.
These notifications are sent when an agent replies to a customer's issue. Your customers can click on these banners to go straight into the conversation screen.
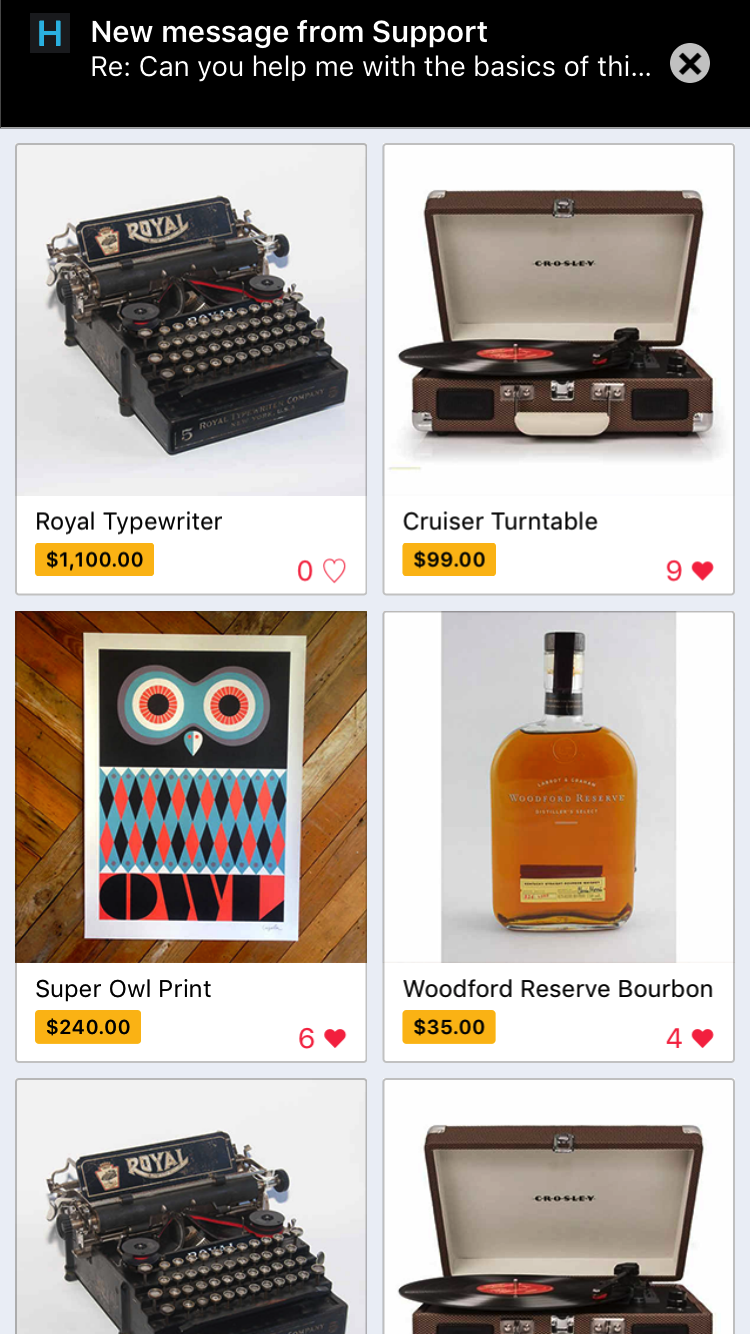
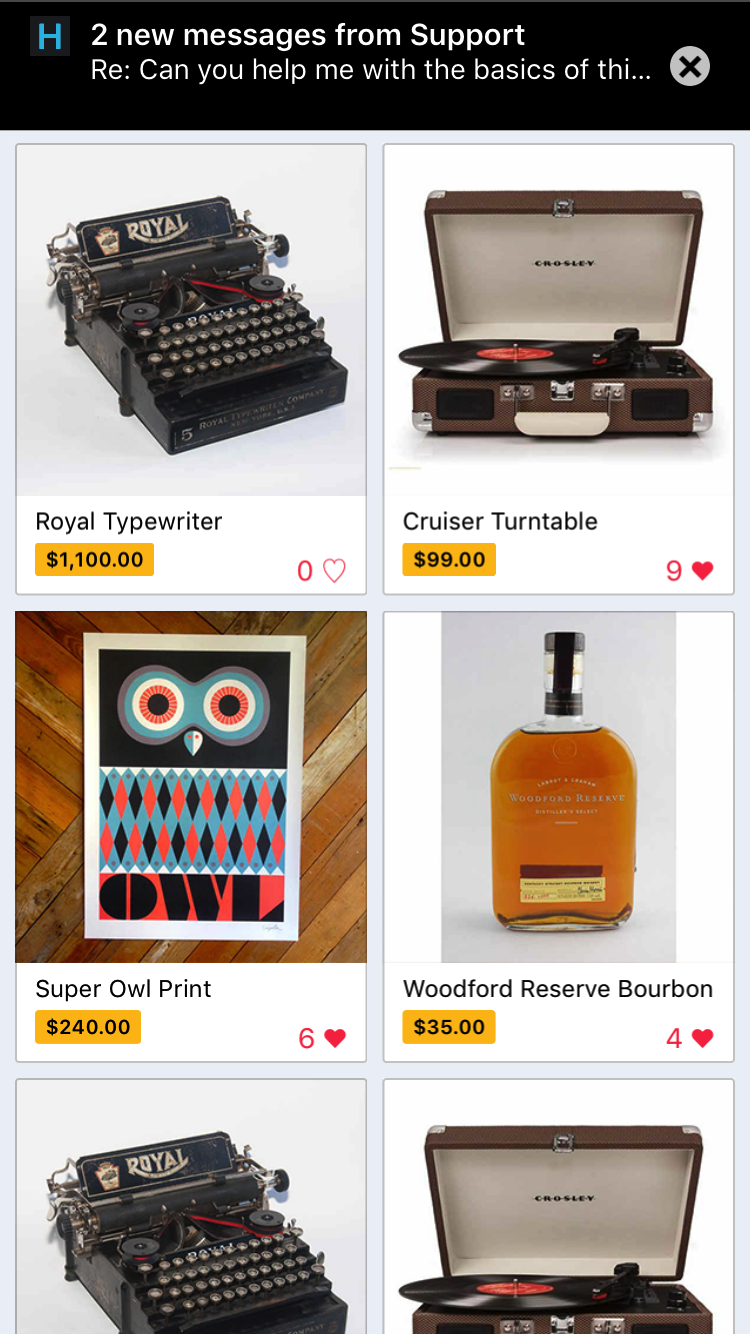
Configuring In-app notifications
The Helpshift install call supports flags for configuring the SDK's behaviour.
Currently we support one flag i.e enableInAppNotification
or you can
use macro HS_ENABLE_IN_APP_NOTIFICATION
in HelpshiftCocos2dx.h
file.
Enabling/Disabling In-app notifications
Flag | enableInAppNotification |
Values | "yes" or "no" (CCString) |
Default | "yes" |
If you do not want the in-app notifications support provided by the
Helpshift SDK, please set this flag to "no"
. The default value of this
flag is "yes"
i.e in-app notifications will be enabled.
Read more about in-app notifications in the Notifications section.
Example:
- Cocos2d-x 3.x
- Cocos2d-x 2.x
ValueMap config;
config[HS_ENABLE_IN_APP_NOTIFICATION] = Value("yes");
HelpshiftCocos2dx::install("apikey",
"domain.helpshift.com",
"appId",
config);
cocos2d::CCDictionary *config = new cocos2d::CCDictionary();
config->setObject(new CCString("yes"), HS_ENABLE_IN_APP_NOTIFICATION);
HelpshiftCocos2dx::install("apikey",
"domain.helpshift.com",
"appId",
config);
Pausing In-app notifications
If you have enabled in-app notifications, use the API pauseDisplayOfInAppNotification
to pause/resume the notifications. When true
is passed to this method display of in-app notifications is paused even if they arrive. When you pass a false
, in-app notifications start displaying.
Example:
HelpshiftCocos2dx::pauseDisplayOfInAppNotification(true);
Showing notification count when replies are sent to the user
This API takes a function pointer as an argument and calls that function with the count of unread messages as its parameter. The first parameter (isRemote) passed to the API requestUnreadMessagesCount will decide if the count is to be fetched remotely.
void didReceiveUnreadMessagesCount(int count) {
printf("Unread message count: %d\n", count);
}
HelpshiftCocos2dx::requestUnreadMessagesCount(true, didReceiveUnreadMessagesCount);
Delegates and Notification Count
If you now call the method
HelpshiftCocos2dx::requestUnreadMessagesCount(true, didReceiveUnreadMessagesCount);
it will return a notification count from server in thedidReceiveUnreadMessagesCount(int count)
delegate method. The notification count here can be fetched either from the cache or from the Helpshift servers, the count from Helpshift’s servers is rate limited and it returns the value only if a subsequent call is made to the API, after the reset timeout or when the user just closes the chat screen (whichever is earlier). For an active issue, the reset timeout is 1 minute and 5 minutes for inactive issues.If you want to retrieve the current notification count stored locally, you can call the same method with the parameter set to false,
HelpshiftCocos2dx::requestUnreadMessagesCount(false, didReceiveUnreadMessagesCount);
. In this case, SDK returns the count of unread messages available locally in thedidReceiveUnreadMessagesCount(int count)
delegate method.Locally saved unread message count are useful for saving an additional network call.
If end user has turned off push notifications, locally saved count may be inaccurate. In that case, use count from server.